springboot jar包 调接口执行 linux命令
时间: 2024-09-27 13:10:00 浏览: 41
在Spring Boot应用中,如果你想要通过调用接口的方式来执行Linux命令,通常的做法是利用`java.lang.Runtime.getRuntime()` 或者第三方库如`org.springframework.shell.core.CommandLineRunner`。以下是一个简单的步骤:
1. **创建接口**:
创建一个接口,比如`ShellCommandService`, 定义一个执行Linux命令的方法,例如:
```java
public interface ShellCommandService {
String executeCommand(String command);
}
```
2. **实现接口**:
实现该接口,利用Runtime或第三方库来执行命令:
```java
@Component
class LinuxCommandExecutor implements ShellCommandService {
@Override
public String executeCommand(String command) {
try {
Process process = Runtime.getRuntime().exec(command);
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
StringBuilder output = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
output.append(line).append("\n");
}
return output.toString();
} catch (IOException e) {
throw new RuntimeException("Failed to execute command", e);
}
}
}
```
3. **在API接口中调用**:
在需要调用Linux命令的地方,注入`ShellCommandService`并调用其方法,例如在一个Controller里:
```java
@RestController
public class MyController {
private final ShellCommandService commandService;
@Autowired
public MyController(ShellCommandService commandService) {
this.commandService = commandService;
}
@GetMapping("/execute-command")
public ResponseEntity<String> executeLinuxCommand(@RequestParam String command) {
String result = commandService.executeCommand(command);
// 返回结果或处理异常
return ResponseEntity.ok(result);
}
}
```
当你访问`/execute-command?command=your-linux-command`这样的URL时,Spring Boot会自动调用相应的接口,并将Linux命令作为参数传递给`executeCommand`方法。
阅读全文
相关推荐
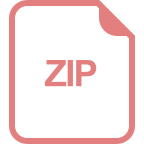
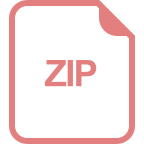
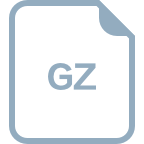
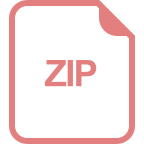
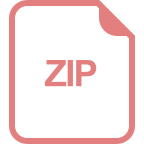
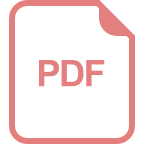
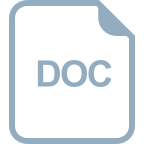
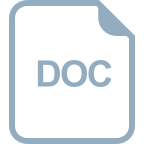
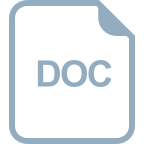
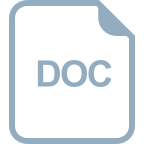
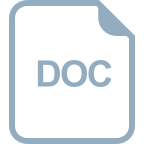
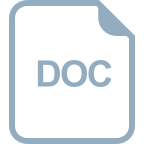
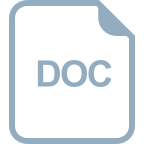
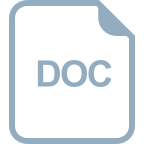
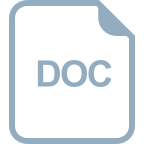
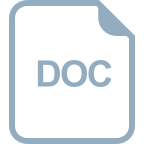
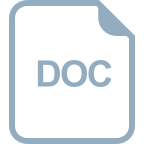
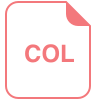
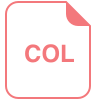