用C语言写关于:线性表顺序存储结构下基本操作的实现(初始化、赋值、取值、查找、插入、删除、两个非递减有序顺序表的归并等),的代码。
时间: 2024-10-16 15:16:21 浏览: 43
在C语言中,线性表的顺序存储结构通常使用数组来实现。以下是基本操作的代码示例:
```c
#include <stdio.h>
// 定义单个元素结构体(如果有复杂的数据结构,可以替换)
typedef struct Node {
int data; // 存储数据的变量
struct Node* next; // 指向下一个节点的指针
} Node;
// 初始化顺序表
Node* createList(int n) {
Node* head = (Node*)malloc(sizeof(Node)); // 分配头节点空间
if (!head) {
printf("Memory allocation failed.\n");
return NULL;
}
head->data = 0; // 非初始化状态下设为0或其他默认值
head->next = NULL;
for (int i = 1; i < n; i++) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed at index %d.\n", i);
free(head); // 如果失败,释放已分配的内存
return NULL;
}
newNode->data = i;
newNode->next = head->next;
head->next = newNode;
}
return head;
}
// 插入操作
void insert(Node** head, int value, int pos) {
if (pos <= 0 || pos > size(*head)) {
printf("Invalid position.\n");
return;
}
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = (*head)->next;
for (int i = 1; i < pos; i++) {
(*head)->next = (*head)->next->next;
}
(*head)->next = newNode;
}
// 删除操作(这里仅提供删除第一个节点的例子)
void deleteFirst(Node** head) {
if (*head == NULL) {
printf("List is empty.\n");
return;
}
Node* temp = *head;
*head = (*head)->next;
free(temp);
}
// 查找操作
int search(Node* head, int key) {
Node* current = head;
while (current != NULL) {
if (current->data == key)
return 1; // 找到元素,返回1
current = current->next;
}
return 0; // 未找到元素,返回0
}
// 归并两个非递减有序表
Node* mergeLists(Node* list1, Node* list2) {
Node* result = NULL;
if (list1 == NULL)
return list2;
else if (list2 == NULL)
return list1;
if (list1->data <= list2->data) {
result = list1;
result->next = mergeLists(list1->next, list2);
} else {
result = list2;
result->next = mergeLists(list1, list2->next);
}
return result;
}
// 显示顺序表内容
void printList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
int n = 5;
Node* list = createList(n);
// ...其他操作...
return 0;
}
```
以上代码展示了顺序表的一些基本操作,包括初始化、插入、删除、查找以及两个有序表的归并。注意,这个版本只实现了简单的插入和删除,对于更复杂的插入和删除场景,可能需要额外处理边界情况。
阅读全文
相关推荐
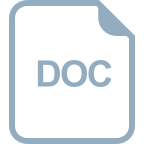
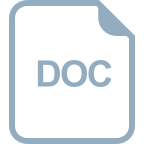
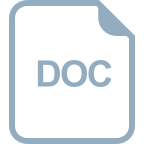



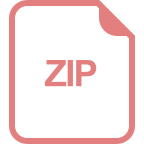
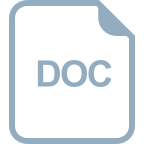
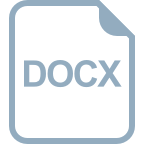
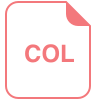
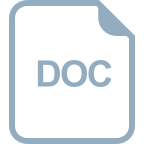
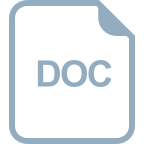
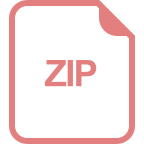
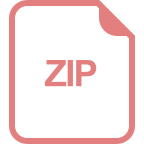
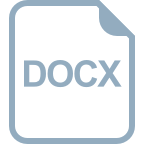