用java画一个动画版的圣诞树
时间: 2023-12-25 13:29:28 浏览: 104
以下是一个用Java绘制动画版圣诞树的示例代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ChristmasTreeAnimation extends JPanel implements ActionListener {
private int treeHeight;
private int frameCount;
private Timer timer;
public ChristmasTreeAnimation(int treeHeight) {
this.treeHeight = treeHeight;
this.frameCount = 0;
this.timer = new Timer(100, this);
this.timer.start();
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
// 绘制背景
g2d.setColor(Color.BLACK);
g2d.fillRect(0, 0, getWidth(), getHeight());
// 绘制圣诞树
drawChristmasTree(g2d, getWidth() / 2, getHeight() - 50, treeHeight, frameCount);
// 绘制文字
g2d.setColor(Color.WHITE);
g2d.setFont(new Font("Arial", Font.BOLD, 20));
g2d.drawString("Merry Christmas!", getWidth() / 2 - 80, getHeight() - 20);
}
private void drawChristmasTree(Graphics2D g2d, int x, int y, int height, int frame) {
int trunkHeight = height / 5;
int trunkWidth = height / 10;
int treeTopHeight = height - trunkHeight;
// 绘制树冠
g2d.setColor(new Color(34, 139, 34));
g2d.fillPolygon(new int[]{x - height / 2, x + height / 2, x}, new int[]{y - treeTopHeight, y - treeTopHeight, y}, 3);
// 绘制树干
g2d.setColor(new Color(139, 69, 19));
g2d.fillRect(x - trunkWidth / 2, y, trunkWidth, trunkHeight);
// 绘制装饰品
int ornamentSize = height / 10;
int ornamentSpacing = height / 8;
int ornamentY = y - treeTopHeight + ornamentSize / 2;
int ornamentX = x - height / 2 + ornamentSize / 2;
for (int i = 0; i < height / ornamentSpacing; i++) {
for (int j = 0; j < i + 1; j++) {
if ((frame / 10 + i + j) % 2 == 0) {
g2d.setColor(Color.RED);
} else {
g2d.setColor(Color.YELLOW);
}
g2d.fillOval(ornamentX + j * ornamentSpacing, ornamentY + i * ornamentSpacing, ornamentSize, ornamentSize);
}
}
}
@Override
public void actionPerformed(ActionEvent e) {
frameCount++;
repaint();
}
public static void main(String[] args) {
JFrame frame = new JFrame("Christmas Tree Animation");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(800, 600);
frame.setLocationRelativeTo(null);
ChristmasTreeAnimation animation = new ChristmasTreeAnimation(300);
frame.add(animation);
frame.setVisible(true);
}
}
```
这段代码使用了Java的Swing库来创建一个窗口,并在窗口中绘制动画版的圣诞树。圣诞树的形状由三角形和矩形组成,装饰品使用圆形表示。通过定时器控制每一帧的绘制,使得装饰品呈现动画效果。
阅读全文
相关推荐
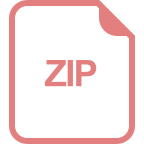
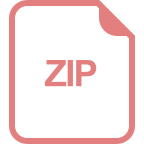
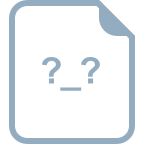
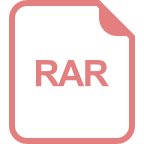
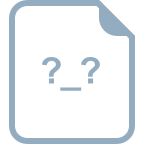
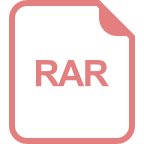
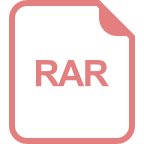
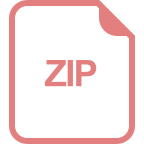
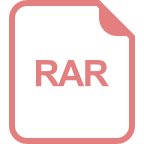
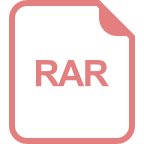
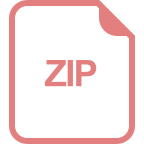
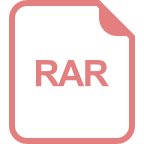
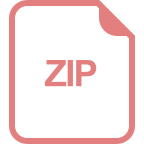
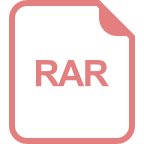
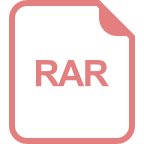
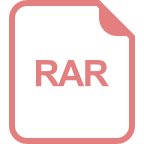
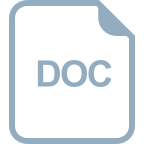