编写一个程序,搜索一个int类型的数字文件,并将最大和最小的数字写到屏幕上。该文件只包含由空格或换行符分隔的int类型的数字。用C++
时间: 2024-09-29 11:04:05 浏览: 38
为了编写一个C++程序来搜索整数文件并找到其中的最大和最小值,你可以采用以下步骤:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <limits> // 使用std::numeric_limits获取INT_MAX和INT_MIN
// 函数用来读取单个整数
int readInt(ifstream& file) {
int num;
if (file >> num) {
return num;
} else {
throw runtime_error("Invalid integer found or end of file reached");
}
}
// 主函数
int main() {
try {
// 打开文件
ifstream inputFile("input.txt", ios::in);
// 检查文件是否打开成功
if (!inputFile) {
cerr << "Error opening the file!" << endl;
return 1;
}
vector<int> numbers; // 存储从文件中读取的数字
int num;
while (inputFile >> num) { // 逐行读取直到文件结束
numbers.push_back(num);
}
// 关闭文件
inputFile.close();
// 如果有数字,则找出最大和最小值
if (!numbers.empty()) {
int maxNum = *max_element(numbers.begin(), numbers.end());
int minNum = *min_element(numbers.begin(), numbers.end());
cout << "The maximum number is: " << maxNum << endl;
cout << "The minimum number is: " << minNum << endl;
} else {
cout << "No valid integers found in the file." << endl;
}
} catch (const exception& e) {
cerr << "Error: " << e.what() << endl;
}
return 0;
}
```
这个程序首先尝试打开名为"input.txt"的文件,然后逐行读取并存储整数。如果遇到无效的输入或文件无法打开,它会抛出异常。最后,程序查找并打印出文件中的最大和最小整数值。
阅读全文
相关推荐







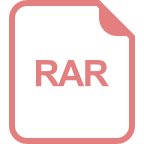



