可以帮我写python程序转为exe文件的代码嘛
时间: 2023-05-26 15:01:52 浏览: 101
当然可以。下面是使用PyInstaller库将Python程序转换为.exe文件的示例代码。
```python
# 导入PyInstaller库
import os
import PyInstaller.__main__
# 设置文件路径
file_path = "example.py"
# 打包exe文件
PyInstaller.__main__.run([
'--name=%s' % "example", # 指定生成的exe文件名称
'--onefile', # 生成单个exe文件
file_path # 要打包的Python脚本路径
])
# 删除多余文件
os.unlink("example.spec") # 删除打包配置文件
os.rename("dist/example.exe", "example.exe") # 将生成的exe文件重命名并移动到当前目录下
os.rmdir("build") # 删除生成的build文件夹
os.rmdir("__pycache__") # 删除生成的__pycache__文件夹
```
你只需要将上述代码中的`file_path`变量设置为你要转换为.exe文件的Python脚本的路径,然后运行这段代码即可将Python脚本转换为.exe文件。在这个例子中,我们将生成的.exe文件命名为`example.exe`。
相关问题
能帮我写一下python程序转为exe文件的代码么
当然可以,以下是将Python脚本转换为可执行文件(exe)的示例代码:
```python
# 导入相关库
from cx_Freeze import setup, Executable
# 设置参数
setup(
name = "MyPythonProgram", # 程序名称
version = "1.0", # 程序版本号
description = "My Python Program Description", # 程序描述
executables = [Executable("main.py")] # 需要打包的Python脚本,可以有多个
)
```
其中,`cx_Freeze` 是一种 Python 的第三方库,它可以将 Python 脚本打包成可执行文件。
在上述代码中,我们使用 `setup` 函数来设置程序的名称、版本号、描述以及需要打包的 Python 脚本。 `Executable` 函数则是指定 Python 脚本的入口。
将以上代码保存为 `setup.py` 文件,然后在命令行中使用如下命令将程序打包:
```
python setup.py build
```
执行这个命令后,cx_Freeze 会自动将指定的 Python 脚本以及相关依赖打包为一个可执行文件。打包完成后,可执行文件位于 `./build/exe.win-amd64-3.6` 目录下(注:这里的路径是根据我的环境自动生成的,具体路径可能会不同)。
希望以上代码对你有所帮助。
将python代码转为c代码
将Python代码转换为C代码可以提高代码的执行效率和速度。由于Python是一门解释型语言,相对于编译型语言如C语言而言,Python的执行速度较慢。因此,在某些情况下,将Python代码转换为C代码可以提高程序的性能。
以下是两种将Python代码转换为C代码的方法:
1. 使用Cython[^1]:
Cython是一种将Python代码转换为C代码的工具。它允许你在Python代码中使用静态类型声明,从而提高代码的执行效率。使用Cython,你可以将Python代码编译为C代码,并将其与C语言库进行链接。这样可以获得接近原生C代码的执行速度。
2. 使用Swig:
Swig是一种将Python代码转换为C代码的工具。它允许你使用简单的接口描述文件将Python代码转换为C代码,并生成Python和C之间的接口。通过使用Swig,你可以将Python代码转换为C代码,并在C语言环境中使用它。
这些工具可以帮助你将Python代码转换为C代码,从而提高代码的执行效率和速度。
阅读全文
相关推荐
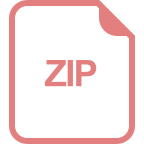
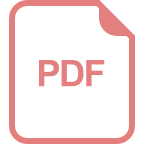
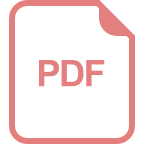
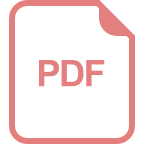
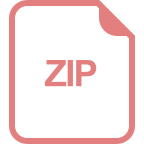
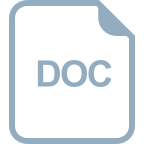
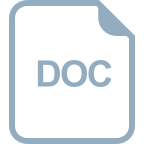
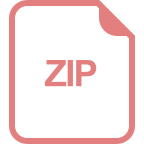
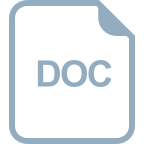






