使用springboot框架搭建一个rabbitmq的demo
时间: 2023-08-04 18:13:35 浏览: 58
好的,下面是一个使用 Spring Boot 框架搭建 RabbitMQ 的示例:
1. 首先在 `pom.xml` 中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
```
2. 在 `application.yml` 中添加 RabbitMQ 的配置信息:
```yml
spring:
rabbitmq:
host: localhost
port: 5672
username: guest
password: guest
```
3. 创建一个简单的消息生产者:
```java
@Component
public class SimpleProducer {
@Autowired
private RabbitTemplate rabbitTemplate;
public void send(String message) {
rabbitTemplate.convertAndSend("simple.queue", message);
}
}
```
4. 创建一个简单的消息消费者:
```java
@Component
public class SimpleConsumer {
@RabbitListener(queues = "simple.queue")
public void receive(String message) {
System.out.println("Received message: " + message);
}
}
```
5. 最后,在主类中运行生产者发送消息:
```java
@SpringBootApplication
public class RabbitmqDemoApplication {
public static void main(String[] args) {
SpringApplication.run(RabbitmqDemoApplication.class, args);
SimpleProducer producer = context.getBean(SimpleProducer.class);
producer.send("Hello, RabbitMQ!");
}
}
```
以上就是一个简单的 RabbitMQ 的 demo,其中 `simple.queue` 是消息队列的名称。你可以按照上述步骤进行实现,当然还有更多高级用法可以参考 RabbitMQ 官方文档。
相关推荐
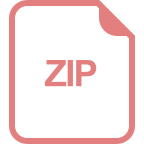














