用C# 写一个点到直线求最短距离的程序
时间: 2024-09-15 15:08:10 浏览: 57
在C#中,我们可以使用向量和数学运算来计算点到直线的距离。首先,我们需要定义一个点和一条直线。假设直线由两个点定义(P1和P2),而我们要找的是点Q到这条直线的距离。
下面是一个简单的示例程序:
```csharp
using System;
using System.Drawing;
class Program
{
// 点类
public class Point
{
public double X { get; set; }
public double Y { get; set; }
public Point(double x, double y)
{
X = x;
Y = y;
}
}
// 直线类,这里假设通过两点P1和P2
public class Line
{
public Point P1 { get; set; }
public Point P2 { get; set; }
public Line(Point p1, Point p2)
{
P1 = p1;
P2 = p2;
}
// 计算斜率和y轴截距
public double Slope()
{
return (P2.Y - P1.Y) / (P2.X - P1.X);
}
public double Intercept()
{
if (P1.X == P2.X)
throw new ArgumentException("Vertical line not allowed");
return P1.Y - Slope() * P1.X;
}
}
// 点到直线距离函数
public static double DistanceToLine(Point point, Line line)
{
double numerator = Math.Abs(line.P1.Y - point.Y - line.Intercept()) * Math.Cos(Math.Atan2(point.X - line.P1.X, line.Slope()));
double denominator = Math.Sqrt(Math.Pow(line.P2.X - line.P1.X, 2) + Math.Pow(line.P2.Y - line.P1.Y, 2));
return numerator / denominator;
}
static void Main(string[] args)
{
Point Q = new Point(5, 10); // 要寻找距离的点
Line line = new Line(new Point(0, 0), new Point(10, 5)); // 定义直线
try
{
double distance = DistanceToLine(Q, line);
Console.WriteLine($"Point ({Q.X}, {Q.Y}) is closest to the line at a distance of {distance:F2}.");
}
catch (ArgumentException ex)
{
Console.WriteLine(ex.Message);
}
}
}
```
在这个程序中,`DistanceToLine`函数计算了点Q到直线的垂线距离,然后返回这个值。注意,垂直线的情况需要特殊处理,因为传统的点到直线距离公式在此不适用。
阅读全文
相关推荐
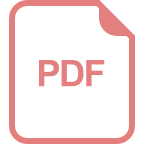
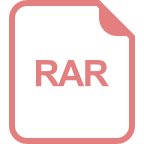
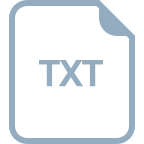
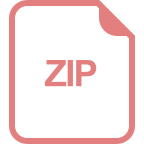
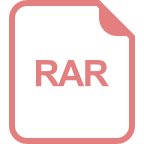
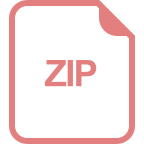
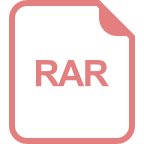
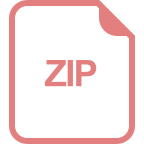
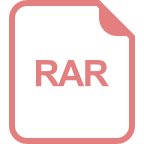
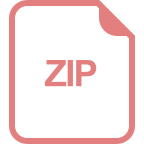
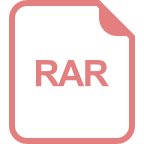
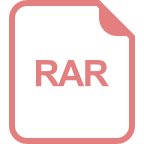