下列给定程序函数fun的功能是:统计子字符串substr在字符串str中出现的次数。例如,若字符串aaas lkaaas,子字符串为as,则应输出2。
时间: 2024-09-21 16:10:11 浏览: 64
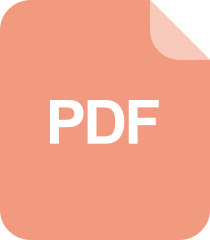
PHP中substr_count()函数获取子字符串出现次数的方法
函数`fun`的主要功能是通过遍历字符串`str`,查找子字符串`substr`在`str`中出现的位置,并计算子字符串出现的总次数。它通常会采用循环结构,每次搜索子字符串开始位置,如果找到匹配的部分,计数器加一,然后继续搜索直到字符串结束。这个过程可能会用到字符串处理函数如`find()`或`indexOf()`来定位子字符串。
下面是一个简单的伪代码示例:
```python
def fun(str, substr):
count = 0
start = 0
while True:
index = str.find(substr, start)
if index == -1: # 如果找不到子串,返回当前计数
return count
count += 1 # 找到子串,计数加一
start = index + 1 # 更新搜索起始位置,跳过已找到的部分
# 示例:
str = "aaas lkaaas"
substr = "as"
result = fun(str, substr)
print("子字符串", substr, "在", str, "中出现了", result, "次")
```
阅读全文
相关推荐
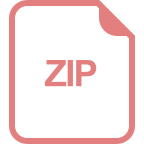
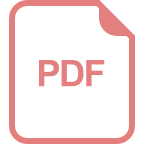
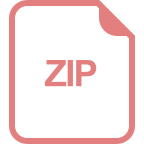
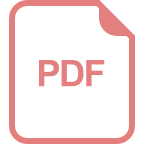
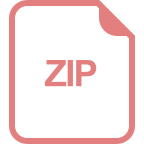
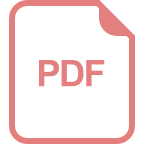
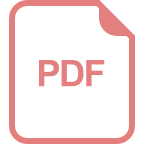
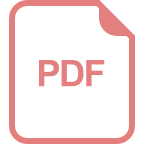
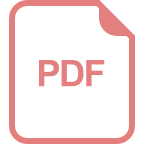
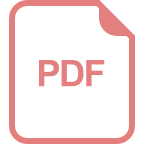
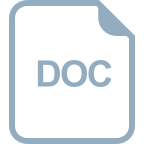
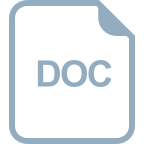
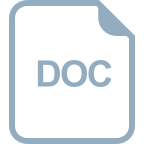
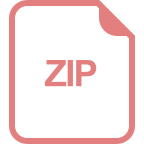
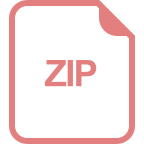
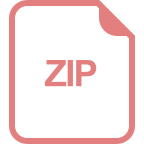
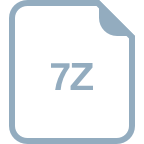