Java建立实体类病人字段有年龄、疾病种类等
时间: 2023-07-10 19:05:52 浏览: 51
可以按照如下方式在Java中建立一个病人实体类:
```
public class Patient {
private int age;
private String diseaseType;
public Patient(int age, String diseaseType) {
this.age = age;
this.diseaseType = diseaseType;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getDiseaseType() {
return diseaseType;
}
public void setDiseaseType(String diseaseType) {
this.diseaseType = diseaseType;
}
}
```
在这个实体类中,我们定义了两个字段,即年龄和疾病种类,并提供了对这两个字段进行读写的方法。在构造函数中,我们接受年龄和疾病种类作为参数,并将它们分别赋值给对应的字段。
相关问题
Java建立实体类医生字段有职业、兴趣等,实体类病人字段有年龄、疾病种类等
可以使用以下代码建立Java实体类Doctor和Patient:
```
// Doctor.java
public class Doctor {
private String profession;
private String interest;
public Doctor(String profession, String interest) {
this.profession = profession;
this.interest = interest;
}
public String getProfession() {
return profession;
}
public void setProfession(String profession) {
this.profession = profession;
}
public String getInterest() {
return interest;
}
public void setInterest(String interest) {
this.interest = interest;
}
}
// Patient.java
public class Patient {
private int age;
private String disease;
public Patient(int age, String disease) {
this.age = age;
this.disease = disease;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getDisease() {
return disease;
}
public void setDisease(String disease) {
this.disease = disease;
}
}
```
以上代码中,Doctor类有两个私有变量profession和interest,对应医生的职业和兴趣。Patient类有两个私有变量age和disease,对应病人的年龄和疾病种类。每个类都有一个构造函数和对应的getter和setter方法。在构造函数中,可以传入初始变量值并进行初始化。getter和setter方法用于获取和设置私有变量值。
Java建立实体类医生字段有医生名称、职业、兴趣等 实体类病人字段有名称、年龄、疾病种类等 一个医生对应多个病人
可以使用Java的面向对象思想,设计医生类和病人类,并在医生类中添加一个存储病人的集合。
下面是一个简单的示例代码:
```java
public class Doctor {
private String name;
private String profession;
private String interest;
private List<Patient> patients = new ArrayList<>();
public Doctor(String name, String profession, String interest) {
this.name = name;
this.profession = profession;
this.interest = interest;
}
public void addPatient(Patient patient) {
patients.add(patient);
}
// 省略getter和setter方法
}
public class Patient {
private String name;
private int age;
private String diseaseType;
public Patient(String name, int age, String diseaseType) {
this.name = name;
this.age = age;
this.diseaseType = diseaseType;
}
// 省略getter和setter方法
}
```
在上述代码中,Doctor类包含了一个List类型的属性patients,用于存储它所管理的病人。同时,Doctor类还包含了一个addPatient方法,用于向patients集合中添加病人。
使用示例代码:
```java
Doctor doctor = new Doctor("张医生", "内科医生", "旅游");
Patient patient1 = new Patient("小明", 20, "感冒");
Patient patient2 = new Patient("小红", 25, "发烧");
doctor.addPatient(patient1);
doctor.addPatient(patient2);
```
在上述示例代码中,我们创建了一个Doctor对象,然后分别创建了两个Patient对象,并通过Doctor的addPatient方法将病人添加到Doctor的patients集合中。
相关推荐
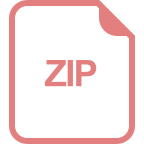
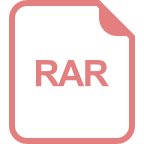












