用java将连接到Hadoop文件系统,并列出给定路径中的所有文件并写入文件或删除文
时间: 2023-12-10 07:38:28 浏览: 34
要连接到Hadoop文件系统,需要使用Hadoop的Java API。以下是一个示例代码,用于列出给定路径中的所有文件并写入文件或删除文件:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.util.ArrayList;
import java.util.List;
public class HadoopFileSystemExample {
private static final String HDFS_URI = "hdfs://localhost:9000";
public static void main(String[] args) throws IOException {
Configuration configuration = new Configuration();
configuration.set("fs.defaultFS", HDFS_URI);
FileSystem fileSystem = FileSystem.get(configuration);
// 列出给定路径中的所有文件
List<String> files = listFiles(fileSystem, "/path/to/files");
// 写入文件
writeToFile(fileSystem, "/path/to/output/file", "Hello World!");
// 删除文件
deleteFile(fileSystem, "/path/to/output/file");
fileSystem.close();
}
private static List<String> listFiles(FileSystem fileSystem, String path) throws IOException {
List<String> files = new ArrayList<>();
Path hdfsPath = new Path(path);
if (fileSystem.exists(hdfsPath)) {
for (org.apache.hadoop.fs.FileStatus fileStatus : fileSystem.listStatus(hdfsPath)) {
if (fileStatus.isFile()) {
files.add(fileStatus.getPath().toString());
}
}
}
return files;
}
private static void writeToFile(FileSystem fileSystem, String path, String content) throws IOException {
Path hdfsPath = new Path(path);
BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(fileSystem.create(hdfsPath)));
writer.write(content);
writer.close();
}
private static void deleteFile(FileSystem fileSystem, String path) throws IOException {
Path hdfsPath = new Path(path);
fileSystem.delete(hdfsPath, false);
}
}
```
在此示例中,我们首先创建了一个Hadoop Configuration对象,并设置了默认的文件系统URI。然后,我们通过调用`FileSystem.get()`方法来获取Hadoop FileSystem实例。接下来,我们使用`listFiles()`方法列出给定路径中的所有文件,使用`writeToFile()`方法将字符串写入文件并使用`deleteFile()`方法删除文件。最后,我们关闭了FileSystem实例。
需要注意的是,上述代码需要引入Hadoop的依赖包,例如:
```xml
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-common</artifactId>
<version>2.7.7</version>
</dependency>
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-hdfs</artifactId>
<version>2.7.7</version>
</dependency>
```
相关推荐
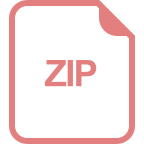
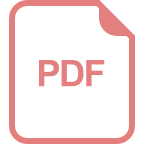
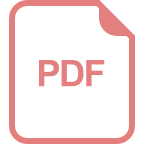














