freq_array = np.linspace(0, sample_rate / 2, num_samples // 2 + 1)
时间: 2024-06-06 13:09:30 浏览: 123
这段代码是用来做什么的?
这段代码是用来生成一个包含频率值的数组,该数组的长度为 num_samples // 2 - 1。其中,sample_rate 是采样率,num_samples 是采样点数。np.linspace(0, sample_rate / 2, num_samples // 2 - 1) 的作用是生成一个从 0 到 sample_rate / 2(采样定理中的奈奎斯特频率)的等距数组,用于表示频率轴。该数组中的元素数量用 num_samples // 2 - 1 来保证只包含正频率分量,因为复数的频率分量是对称的,包括负频率分量。
相关问题
详细解释以下Python代码:import numpy as np import adi import matplotlib.pyplot as plt sample_rate = 1e6 # Hz center_freq = 915e6 # Hz num_samps = 100000 # number of samples per call to rx() sdr = adi.Pluto("ip:192.168.2.1") sdr.sample_rate = int(sample_rate) # Config Tx sdr.tx_rf_bandwidth = int(sample_rate) # filter cutoff, just set it to the same as sample rate sdr.tx_lo = int(center_freq) sdr.tx_hardwaregain_chan0 = -50 # Increase to increase tx power, valid range is -90 to 0 dB # Config Rx sdr.rx_lo = int(center_freq) sdr.rx_rf_bandwidth = int(sample_rate) sdr.rx_buffer_size = num_samps sdr.gain_control_mode_chan0 = 'manual' sdr.rx_hardwaregain_chan0 = 0.0 # dB, increase to increase the receive gain, but be careful not to saturate the ADC # Create transmit waveform (QPSK, 16 samples per symbol) num_symbols = 1000 x_int = np.random.randint(0, 4, num_symbols) # 0 to 3 x_degrees = x_int*360/4.0 + 45 # 45, 135, 225, 315 degrees x_radians = x_degrees*np.pi/180.0 # sin() and cos() takes in radians x_symbols = np.cos(x_radians) + 1j*np.sin(x_radians) # this produces our QPSK complex symbols samples = np.repeat(x_symbols, 16) # 16 samples per symbol (rectangular pulses) samples *= 2**14 # The PlutoSDR expects samples to be between -2^14 and +2^14, not -1 and +1 like some SDRs # Start the transmitter sdr.tx_cyclic_buffer = True # Enable cyclic buffers sdr.tx(samples) # start transmitting # Clear buffer just to be safe for i in range (0, 10): raw_data = sdr.rx() # Receive samples rx_samples = sdr.rx() print(rx_samples) # Stop transmitting sdr.tx_destroy_buffer() # Calculate power spectral density (frequency domain version of signal) psd = np.abs(np.fft.fftshift(np.fft.fft(rx_samples)))**2 psd_dB = 10*np.log10(psd) f = np.linspace(sample_rate/-2, sample_rate/2, len(psd)) # Plot time domain plt.figure(0) plt.plot(np.real(rx_samples[::100])) plt.plot(np.imag(rx_samples[::100])) plt.xlabel("Time") # Plot freq domain plt.figure(1) plt.plot(f/1e6, psd_dB) plt.xlabel("Frequency [MHz]") plt.ylabel("PSD") plt.show(),并分析该代码中QPSK信号的功率谱密度图的特点
这段Python代码的作用是使用ADI Pluto SDR设备生成并传输一个QPSK信号,并将接收到的信号进行功率谱密度分析。下面是对代码的注释:
```
import numpy as np
import adi
import matplotlib.pyplot as plt
# 设置采样率、中心频率和采样点数
sample_rate = 1e6 # Hz
center_freq = 915e6 # Hz
num_samps = 100000 # number of samples per call to rx()
# 连接ADI Pluto SDR设备
sdr = adi.Pluto("ip:192.168.2.1")
sdr.sample_rate = int(sample_rate)
# 配置发送端的参数
sdr.tx_rf_bandwidth = int(sample_rate) # filter cutoff, just set it to the same as sample rate
sdr.tx_lo = int(center_freq)
sdr.tx_hardwaregain_chan0 = -50 # Increase to increase tx power, valid range is -90 to 0 dB
# 配置接收端的参数
sdr.rx_lo = int(center_freq)
sdr.rx_rf_bandwidth = int(sample_rate)
sdr.rx_buffer_size = num_samps
sdr.gain_control_mode_chan0 = 'manual'
sdr.rx_hardwaregain_chan0 = 0.0 # dB, increase to increase the receive gain, but be careful not to saturate the ADC
# 创建发送的QPSK信号
num_symbols = 1000
x_int = np.random.randint(0, 4, num_symbols) # 0 to 3
x_degrees = x_int*360/4.0 + 45 # 45, 135, 225, 315 degrees
x_radians = x_degrees*np.pi/180.0 # sin() and cos() takes in radians
x_symbols = np.cos(x_radians) + 1j*np.sin(x_radians) # this produces our QPSK complex symbols
samples = np.repeat(x_symbols, 16) # 16 samples per symbol (rectangular pulses)
samples *= 2**14 # The PlutoSDR expects samples to be between -2^14 and +2^14, not -1 and +1 like some SDRs
# 启动发送端并发送信号
sdr.tx_cyclic_buffer = True # Enable cyclic buffers
sdr.tx(samples) # start transmitting
# 接收接收端的信号
for i in range (0, 10):
raw_data = sdr.rx() # Receive samples
rx_samples = sdr.rx()
print(rx_samples)
# 停止发送端
sdr.tx_destroy_buffer()
# 计算接收到的信号的功率谱密度
psd = np.abs(np.fft.fftshift(np.fft.fft(rx_samples)))**2
psd_dB = 10*np.log10(psd)
f = np.linspace(sample_rate/-2, sample_rate/2, len(psd))
# 绘制时域图
plt.figure(0)
plt.plot(np.real(rx_samples[::100]))
plt.plot(np.imag(rx_samples[::100]))
plt.xlabel("Time")
# 绘制频域图
plt.figure(1)
plt.plot(f/1e6, psd_dB)
plt.xlabel("Frequency [MHz]")
plt.ylabel("PSD")
plt.show()
```
以上代码生成了一个随机QPSK信号,通过ADI Pluto SDR设备将其传输,并使用Pluto SDR设备接收该信号。接收到的信号进行了功率谱密度分析,并绘制了频域图。
QPSK信号的功率谱密度图的特点是,其频谱表现为四个簇,每个簇对应QPSK信号的一个符号。每个簇的带宽约为基带信号的带宽,且由于使用矩形脉冲,每个簇的带宽之间有一定的重叠。此外,功率谱密度图中还可以看到一些其他频率分量,这些分量可能是由于接收信号中存在其他干扰或噪声导致的。
优化这段import numpy as np import matplotlib.pyplot as plt %config InlineBackend.figure_format='retina' def generate_signal(t_vec, A, phi, noise, freq): Omega = 2*np.pi*freq return A * np.sin(Omega*t_vec + phi) + noise * (2*np.random.random def lock_in_measurement(signal, t_vec, ref_freq): Omega = 2*np.pi*ref_freq ref_0 = 2*np.sin(Omega*t_vec) ref_1 = 2*np.cos(Omega*t_vec) # signal_0 = signal * ref_0 signal_1 = signal * ref_1 # X = np.mean(signal_0) Y = np.mean(signal_1) # A = np.sqrt(X**2+Y**2) phi = np.arctan2(Y,X) print("A=", A, "phi=", phi) # t_vec = np.linspace(0, 0.2, 1001) A = 1 phi = np.pi noise = 0.2 ref_freq = 17.77777 # signal = generate_signal(t_vec, A, phi, noise, ref_freq) # lock_in_measurement(signal, t_vec, ref_freq)
import numpy as np
import matplotlib.pyplot as plt
%config InlineBackend.figure_format='retina'
def generate_signal(t_vec, A, phi, noise, freq):
Omega = 2*np.pi*freq
return A * np.sin(Omega*t_vec + phi) + noise * (2*np.random.random())
def lock_in_measurement(signal, t_vec, ref_freq):
Omega = 2*np.pi*ref_freq
ref_0 = 2*np.sin(Omega*t_vec)
ref_1 = 2*np.cos(Omega*t_vec)
signal_0 = signal * ref_0
signal_1 = signal * ref_1
X = np.mean(signal_0)
Y = np.mean(signal_1)
A = np.sqrt(X**2+Y**2)
phi = np.arctan2(Y,X)
print("A=", A, "phi=", phi)
t_vec = np.linspace(0, 0.2, 1001)
A = 1
phi = np.pi
noise = 0.2
ref_freq = 17.77777
signal = generate_signal(t_vec, A, phi, noise, ref_freq)
lock_in_measurement(signal, t_vec, ref_freq)
阅读全文
相关推荐
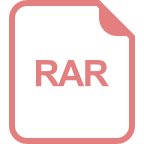
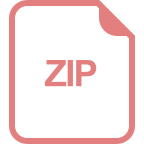
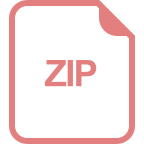












