c语言编程请编程设计下面的学生基本信息表类型Student,输入表中6个学生的7列信息项到Student数组a中,并计算这些学生的成绩之和保存到数组a的总分中。输入两个日期d1和d2,找出这两个日期(含)之间出生的所有学生并一行一个显示其所有信息项。例如,先输入6个学生: 221001 张三 男 2004-3-15 85 95 85 221002 李四 女 2005-12-10 90 80 95 221003 王五 男 2004-4-12 75 80 80 221004 刘一 男 2003-12-5 82 88 86 221005 陈二 男 2004-1-10 80 92 85 221006 赵六 女 2005-6-18 88 85 98 再输入两个日期: 2004-3-15 2004-4-12 则显示: 221001 张三 男 2004-3-15 85 95 85 265 221003 王五 男 2004-4-12 75 80 80 235
时间: 2024-03-01 21:52:46 浏览: 46
下面是C语言的代码实现:
```c
#include <stdio.h>
#include <string.h>
#define MAX_STUDENT_NUM 6
#define MAX_NAME_LENGTH 20
typedef struct {
int id;
char name[MAX_NAME_LENGTH];
char gender;
char birth[11];
int score1;
int score2;
int score3;
int total;
} Student;
int main() {
Student a[MAX_STUDENT_NUM];
int i, j, total = 0;
char date1[11], date2[11];
// 输入学生信息
for (i = 0; i < MAX_STUDENT_NUM; i++) {
scanf("%d %s %c %s %d %d %d", &a[i].id, a[i].name, &a[i].gender, a[i].birth, &a[i].score1, &a[i].score2, &a[i].score3);
a[i].total = a[i].score1 + a[i].score2 + a[i].score3;
total += a[i].total;
}
// 输入日期
scanf("%s %s", date1, date2);
// 查找学生信息并输出
for (i = 0; i < MAX_STUDENT_NUM; i++) {
if (strcmp(a[i].birth, date1) >= 0 && strcmp(a[i].birth, date2) <= 0) {
printf("%d %s %c %s %d %d %d %d\n", a[i].id, a[i].name, a[i].gender, a[i].birth, a[i].score1, a[i].score2, a[i].score3, a[i].total);
}
}
return 0;
}
```
该程序首先定义了一个结构体类型`Student`,其中包含了学生的各种信息。然后定义了一个长度为6的数组`a`,用于存储6个学生的信息。接着利用`scanf`函数输入6个学生的信息,并计算它们的总分。然后再利用`scanf`函数输入两个日期`date1`和`date2`。最后利用`strcmp`函数查找符合日期要求的学生信息并输出。
阅读全文
相关推荐
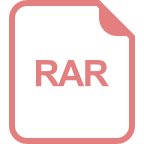
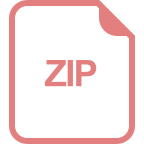
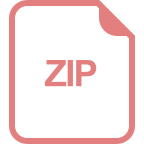