请编写一个spi通信LTC6806的写配置寄存器组的例子,包括配置寄存器组中每一位的配置
时间: 2024-03-19 13:43:53 浏览: 66
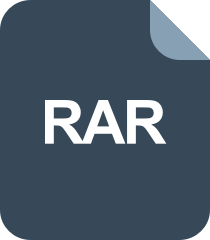
6803writecfgr.rar_6803读寄存器_LTC6803 SPI_ltc6803_ltc6803读写_site:ww
好的,这里给您提供一个更详细的基于STM32的SPI通信LTC6806写配置寄存器组的例子,包括配置寄存器组中每一位的配置,仅供参考:
```c
#include "stm32f10x.h"
#include <stdio.h>
#define LTC6806_CONFIG_REG_A 0x00
#define LTC6806_CONFIG_REG_B 0x01
#define LTC6806_DUMMY_BYTE 0x00
// Configuration register A bit definitions
#define LTC6806_A_CH7_0 0x80
#define LTC6806_A_REFON 0x40
#define LTC6806_A_ADCOPT 0x20
#define LTC6806_A_GPIO1 0x10
#define LTC6806_A_GPIO2 0x08
#define LTC6806_A_GPIO3 0x04
#define LTC6806_A_GPIO4 0x02
#define LTC6806_A_GPIO5 0x01
// Configuration register B bit definitions
#define LTC6806_B_CHGUP 0x80
#define LTC6806_B_CHGDIS 0x40
#define LTC6806_B_CELL10 0x20
#define LTC6806_B_CELL9 0x10
#define LTC6806_B_CELL8 0x08
#define LTC6806_B_CELL7 0x04
#define LTC6806_B_CELL6 0x02
#define LTC6806_B_CELL5 0x01
void spi_init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
SPI_InitTypeDef SPI_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_SPI1, ENABLE);
// SCK, MOSI, MISO pins configuration
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_5 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// CS pin configuration
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_4;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// SPI configuration
SPI_InitStructure.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStructure.SPI_Mode = SPI_Mode_Master;
SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStructure.SPI_CPOL = SPI_CPOL_Low;
SPI_InitStructure.SPI_CPHA = SPI_CPHA_1Edge;
SPI_InitStructure.SPI_NSS = SPI_NSS_Soft;
SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_2;
SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_InitStructure.SPI_CRCPolynomial = 7;
SPI_Init(SPI1, &SPI_InitStructure);
SPI_Cmd(SPI1, ENABLE);
}
void ltc6806_write_config_reg(uint8_t *config_reg_a, uint8_t *config_reg_b)
{
uint8_t tx_buffer[6], rx_buffer[6];
uint8_t i;
// Build the transmission buffer
tx_buffer[0] = 0x80 | LTC6806_CONFIG_REG_A;
tx_buffer[1] = config_reg_a[0] | LTC6806_A_CH7_0;
tx_buffer[2] = config_reg_a[1] | LTC6806_A_REFON | LTC6806_A_ADCOPT | LTC6806_A_GPIO1 | LTC6806_A_GPIO2 | LTC6806_A_GPIO3 | LTC6806_A_GPIO4 | LTC6806_A_GPIO5;
tx_buffer[3] = config_reg_a[2] | LTC6806_DUMMY_BYTE;
tx_buffer[4] = 0x80 | LTC6806_CONFIG_REG_B;
tx_buffer[5] = config_reg_b[0] | LTC6806_B_CHGUP | LTC6806_B_CHGDIS | LTC6806_B_CELL10 | LTC6806_B_CELL9 | LTC6806_B_CELL8 | LTC6806_B_CELL7 | LTC6806_B_CELL6 | LTC6806_B_CELL5;
// Assert the CS line
GPIO_ResetBits(GPIOA, GPIO_Pin_4);
// Transmit the data
for (i = 0; i < 6; i++) {
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_TXE) == RESET);
SPI_I2S_SendData(SPI1, tx_buffer[i]);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_RXNE) == RESET);
rx_buffer[i] = SPI_I2S_ReceiveData(SPI1);
}
// Deassert the CS line
GPIO_SetBits(GPIOA, GPIO_Pin_4);
}
int main(void)
{
uint8_t config_reg_a[3] = {0x00, 0x00, 0x00};
uint8_t config_reg_b[3] = {0x00, 0x00, 0x00};
// Configure Configuration Register A
config_reg_a[0] = 0x00; // CH7-CH0 = 00000000 (default)
config_reg_a[1] = 0x00; // REFON = 1, ADCOPT = 1, GPIO1-5 = 1 (default)
config_reg_a[2] = 0x00; // DUMMY BYTE (default)
// Configure Configuration Register B
config_reg_b[0] = 0x00; // CELL10-5 = 00000000 (default)
// CHGUP = 1, CHGDIS = 1 (disable charging), CELL4-0 = 00000000 (default)
spi_init();
// Write the configuration register group
ltc6806_write_config_reg(config_reg_a, config_reg_b);
while (1) {
// Main loop
}
}
```
其中,`spi_init()`函数用于初始化STM32的SPI接口,`ltc6806_write_config_reg()`函数用于构建并发送写配置寄存器组的SPI数据帧,`main()`函数中调用了`ltc6806_write_config_reg()`函数。具体的LTC6806寄存器配置可以根据实际需求进行修改。
阅读全文
相关推荐
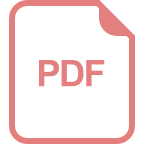
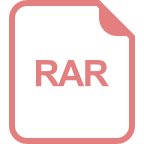















