system verilog中case条件是字符串
时间: 2025-01-03 15:44:10 浏览: 5
SystemVerilog是一种硬件描述语言(HDL),用于设计和验证数字系统。它支持多种数据类型,包括字符串。在SystemVerilog中,你可以使用`case`结构处理字符串,但与常规整数或枚举类型的`case`不同,处理字符串需要借助其他机制。
在`case`语句中处理字符串通常会涉及到`string`类型,并结合`match`操作符来比较。例如:
```systemverilog
reg [7:0] input_string = "Hello";
integer idx;
always @(*) begin
case (input_string)
"Hello": idx = 0;
"World": idx = 1; // 如果输入为"World", idx将等于1
default: idx = -1; // 表示未找到匹配
endcase
$display("String match: %d", idx);
end
```
在这个例子中,`input_string`是一个字符串变量,`case`结构根据`input_string`的内容选择相应的`idx`值。需要注意的是,字符串匹配通常是基于逐字符的比较,而不是像整数那样精确匹配整个串。
相关问题
verilog串行输入检测器
### Verilog 实现串行输入检测器
#### 1. 设计概述
在FPGA中实现串行输入检测器的关键在于理解并遵循串行通信协议的数据帧格式和波特率设置。通过使用Verilog HDL,可以在任意引脚上实现串口功能,并确保数据的正确接收。
#### 2. 数据帧结构
典型的串行数据帧由起始位、数据位、奇偶校验位(可选)以及停止位组成。每种配置可能有所不同,因此需要根据具体应用需求进行调整[^2]。
#### 3. 波特率计算
波特率决定了每位发送的时间间隔。通常情况下,可以通过分频器来生成所需的时钟周期。例如,在9600bps下,如果系统时钟频率为50MHz,则每个比特时间应为\( \frac{1}{9600} \approx 104\mu s\)。此时可以利用计数器对系统时钟进行适当分频以匹配该时间段。
#### 4. 接收状态机设计
为了可靠地捕获到完整的字符序列,采用有限状态机(FSM)模型是一种常见做法。下面给出了一段简化版的状态转移逻辑:
```verilog
module uart_rx #(
parameter CLK_FREQ = 50_000_000, // System clock frequency (Hz)
parameter BAUD_RATE = 9600 // Baud rate of UART communication
)(
input wire clk,
input wire rst_n,
input wire rx_in,
output reg [7:0] data_out,
output reg valid_data
);
localparam integer BITS_PER_CHAR = 8;
localparam real BIT_PERIOD = 1 / real'(BAUD_RATE);
localparam integer CYCLES_PER_BIT = int'((CLK_FREQ * BIT_PERIOD));
typedef enum logic [2:0] {
IDLE,
START_DETECTED,
SAMPLE_BITS,
STOP_CHECKED
} state_t;
state_t current_state, next_state;
reg [$clog2(CYCLES_PER_BIT)-1:0] bit_counter;
reg [BITS_PER_CHAR-1:0] shift_reg;
integer i;
always_ff @(posedge clk or negedge rst_n) begin : proc_current_state
if (!rst_n) begin
current_state <= IDLE;
end else begin
current_state <= next_state;
end
end
// State transition and actions
always_comb begin : proc_next_state_and_actions
case(current_state)
IDLE: begin
if (~rx_in) begin
next_state = START_DETECTED;
bit_counter = 'd0;
shift_reg = 'b0;
valid_data = 1'b0;
end else begin
next_state = IDLE;
end
end
START_DETECTED: begin
if(bit_counter == CYCLES_PER_BIT/2 - 1)begin
next_state = SAMPLE_BITS;
bit_counter = 'd0;
end else begin
next_state = START_DETECTED;
bit_counter += 1;
end
end
SAMPLE_BITS: begin
if(i < BITS_PER_CHAR && bit_counter == CYCLES_PER_BIT - 1) begin
shift_reg[i] = rx_in;
i++;
bit_counter = 'd0;
next_state = SAMPLE_BITS;
end else if(i >= BITS_PER_CHAR){
next_state = STOP_CHECKED;
}else{
next_state = SAMPLE_BITS;
bit_counter += 1;
}
end
STOP_CHECKED: begin
if(rx_in & bit_counter == CYCLES_PER_BIT - 1) begin
data_out = shift_reg;
valid_data = 1'b1;
next_state = IDLE;
end else if(!rx_in | bit_counter != CYCLES_PER_BIT - 1){
next_state = STOP_CHECKED;
bit_counter += 1;
} else {
next_state = IDLE;
}
end
endcase
end
endmodule
```
此模块实现了基本的功能,包括但不限于:
- **IDLE**: 等待低电平表示开始;
- **START_DETECTED**: 当检测到下降沿后等待半个比特宽度再进入采样阶段;
- **SAMPLE_BITS**: 对每一位按照设定好的定时读取其值直到完成整个字节;
- **STOP_CHECKED**: 验证结束标志是否有效;
上述代码展示了如何构建一个简单的UART接收端,能够处理标准ASCII码范围内的单个字符。实际项目中还需要考虑更多细节如错误处理机制等.
阅读全文
相关推荐



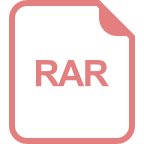










