java+对图像进行直线检测_Opencv Hough算法实现图片中直线检测
时间: 2023-11-28 10:47:49 浏览: 32
使用OpenCV中的HoughLines函数可以实现直线检测。以下是Java代码示例:
```java
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.core.MatOfFloat;
import org.opencv.core.MatOfPoint;
import org.opencv.core.Point;
import org.opencv.core.Scalar;
import org.opencv.core.Size;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
import java.util.ArrayList;
import java.util.List;
public class HoughLinesExample {
public static void main(String[] args) {
// Load the image
Mat src = Imgcodecs.imread("input.jpg");
// Convert the image to grayscale
Mat gray = new Mat();
Imgproc.cvtColor(src, gray, Imgproc.COLOR_BGR2GRAY);
// Perform edge detection using Canny
Mat edges = new Mat();
Imgproc.Canny(gray, edges, 50, 150, 3, false);
// Perform HoughLines transformation
Mat lines = new Mat();
Imgproc.HoughLines(edges, lines, 1, Math.PI / 180, 150);
// Draw the detected lines on the original image
List<MatOfPoint> contours = new ArrayList<>();
for (int i = 0; i < lines.rows(); i++) {
double[] data = lines.get(i, 0);
double rho = data[0];
double theta = data[1];
double a = Math.cos(theta);
double b = Math.sin(theta);
double x0 = a * rho;
double y0 = b * rho;
Point pt1 = new Point(x0 + 1000 * (-b), y0 + 1000 * (a));
Point pt2 = new Point(x0 - 1000 * (-b), y0 - 1000 * (a));
Imgproc.line(src, pt1, pt2, new Scalar(0, 0, 255), 3, Imgproc.LINE_AA, 0);
}
// Display the result
HighGui.imshow("Detected Lines", src);
HighGui.waitKey();
System.exit(0);
}
}
```
需要注意的是,这里使用的是HoughLines函数而不是HoughLinesP函数。HoughLines函数可以检测出所有直线,而HoughLinesP函数只会检测出部分直线。
相关推荐











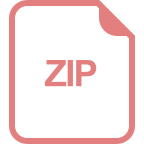