Java将一个list集合写入到excel中并且对关键字进行标红处理的demo
时间: 2024-05-07 17:17:54 浏览: 119
以下是一个示例代码,可以将一个list集合写入到excel中,并且对关键字进行标红处理:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.List;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.Font;
import org.apache.poi.ss.usermodel.IndexedColors;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelWriterDemo {
public static void writeToExcel(List<String> list, String keyword, String filePath) throws IOException {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sheet1");
// 设置关键字样式
Font font = workbook.createFont();
font.setColor(IndexedColors.RED.getIndex());
CellStyle style = workbook.createCellStyle();
style.setFont(font);
// 写入数据
int rowIndex = 0;
for (String item : list) {
Row row = sheet.createRow(rowIndex++);
String[] cells = item.split(",");
int cellIndex = 0;
for (String cell : cells) {
row.createCell(cellIndex++).setCellValue(cell);
}
// 如果该行包含关键字,则对关键字进行标红处理
if (item.contains(keyword)) {
for (int i = 0; i < cells.length; i++) {
row.getCell(i).setCellStyle(style);
}
}
}
// 输出到文件
try (FileOutputStream outputStream = new FileOutputStream(filePath)) {
workbook.write(outputStream);
}
}
}
```
使用方法如下:
```java
List<String> list = Arrays.asList("apple,red,fruit", "banana,yellow,fruit", "carrot,orange,vegetable");
String keyword = "fruit";
String filePath = "output.xlsx";
ExcelWriterDemo.writeToExcel(list, keyword, filePath);
```
以上代码会将list中的数据写入到名为"output.xlsx"的Excel文件中,在该Excel文件中,如果某一行包含关键字"fruit",则该行的所有单元格的字体颜色会被标红。
阅读全文
相关推荐
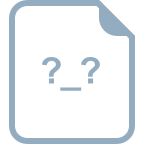
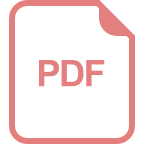
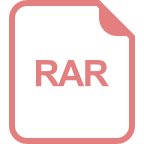
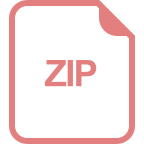
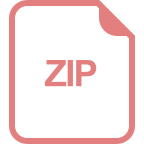
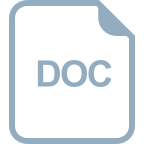
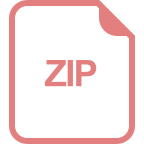
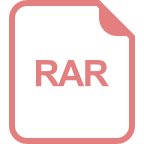
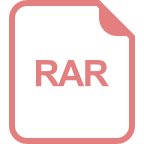
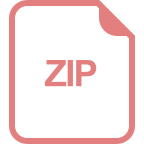
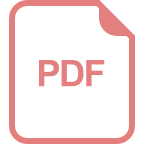
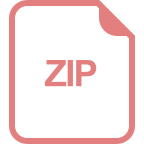
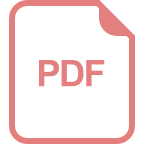
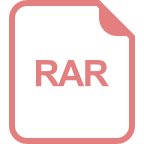