wordcloud = WordCloud(width=800, height=400, background_color='white').generate(news_content)
时间: 2024-03-07 10:52:52 浏览: 178
这行代码使用了 Python 的 wordcloud 库,生成了一个指定宽度、高度和背景颜色的词云图,并将新闻内容作为输入数据。其中,width 和 height 分别指定词云图的宽度和高度,background_color 指定背景颜色,默认为黑色。news_content 变量应该是包含新闻内容的字符串。生成的词云图可以保存为图片或者直接显示在屏幕上。
相关问题
Traceback (most recent call last): File "C:/Users/00/Desktop/pachong/main.py", line 146, in <module> wordcloud = WordCloud(width=800, height=400, background_color='white').generate(news_content) File "C:\Users\00\anaconda3\lib\site-packages\wordcloud\wordcloud.py", line 639, in generate return self.generate_from_text(text) File "C:\Users\00\anaconda3\lib\site-packages\wordcloud\wordcloud.py", line 621, in generate_from_text self.generate_from_frequencies(words) File "C:\Users\00\anaconda3\lib\site-packages\wordcloud\wordcloud.py", line 410, in generate_from_frequencies raise ValueError("We need at least 1 word to plot a word cloud, " ValueError: We need at least 1 word to plot a word cloud, got 0.
这个错误通常是由于提供的文本数据为空导致的。例如,在Python中,下面的代码会导致此错误:
```
from wordcloud import WordCloud
text = ""
wordcloud = WordCloud().generate(text)
```
要解决这个问题,需要确保提供的文本数据不为空。例如,可以从文件中读取文本数据,或者从数据库中检索文本数据。以下是一个示例:
```
from wordcloud import WordCloud
with open("text_file.txt", "r") as file:
news_content = file.read()
if len(news_content) > 0:
wordcloud = WordCloud(width=800, height=400, background_color='white').generate(news_content)
else:
print("Error: No text data found.")
```
这会避免抛出ValueError异常,并正确地处理不足的文本数据。
从https://www.cqwu.edu.cn/channel_24893_03{}.html系列网站中爬取对应新闻的标题,存放在news.txt文件中,使用jieba和WordCloud库输出对应的词云图片。
为了实现这个任务,你需要遵循以下几个步骤:
1. **网络抓取**:
使用Python的`requests`库来获取网页内容,然后使用`BeautifulSoup`解析HTML,提取新闻标题。这里假设URL的模板是`https://www.cqwu.edu.cn/channel_24893_03{page}.html`,其中`{page}`表示页码。
```python
import requests
from bs4 import BeautifulSoup
# 定义URL模板和初始页码
url_template = "https://www.cqwu.edu.cn/channel_24893_03{}.html"
start_page = 1
end_page = 5 # 根据实际需要调整总页数
titles = []
# 爬取并提取每个页面的标题
for page in range(start_page, end_page + 1):
response = requests.get(url_template.format(page))
soup = BeautifulSoup(response.text, 'html.parser')
news_titles = soup.find_all('h2', class_='title') # 假设标题元素有特定class
for title in news_titles:
titles.append(title.text)
# 将所有标题写入news.txt文件
with open("news.txt", "w", encoding="utf-8") as f:
f.write("\n".join(titles))
```
2. **分词与数据预处理**:
使用`jieba`库对标题进行分词,并去除停用词和数字等无关信息。
```python
import jieba
from collections import Counter
# 加载停用词表
stopwords = set(list(jieba.cut_for_search('', HMM=False))) # 使用默认的停用词表
def preprocess(text):
words = [word.lower() for word in jieba.cut(text) if word.isalpha() and not word in stopwords]
return words
# 对每个标题进行预处理并合并结果
preprocessed_titles = [preprocess(title) for title in titles]
# 合并所有分词后的词汇
all_words = []
for title_words in preprocessed_titles:
all_words.extend(title_words)
```
3. **生成词云**:
使用`wordcloud`库创建词云图。
```python
from wordcloud import WordCloud, ImageColorGenerator
import matplotlib.pyplot as plt
# 绘制词云
wordcloud = WordCloud(font_path='simhei.ttf', background_color='white', width=800, height=600).generate_from_frequencies(Counter(all_words))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.savefig('wordcloud.png')
plt.show()
```
注意:这里的代码示例假设`channel_24893_03{page}.html`结构固定,且标题在`<h2>`标签内,同时使用了`simhei.ttf`字体文件,如果实际网页结构有所不同,你可能需要相应地修改解析部分。另外,`jieba.cut_for_search`是一个快速的分词模式,如果你希望更精确的结果可以使用`jieba.lcut`。记得在运行前检查网络连接、安装必要的库(如`lxml`和`matplotlib`),并替换上述示例中的停用词表路径。
阅读全文
相关推荐
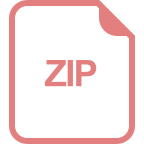
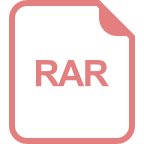






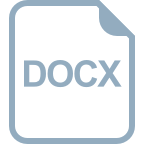
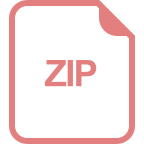