用Java写一个文件上传
时间: 2024-05-09 22:18:59 浏览: 91
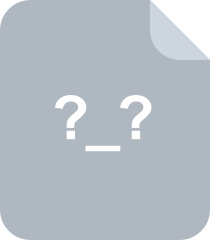
java 文件上传
以下是一个基本的Java文件上传示例:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class FileUploader {
public static void uploadFile(String serverUrl, String filePath) throws IOException {
URL url = new URL(serverUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setDoOutput(true);
connection.setRequestProperty("Content-Type", "multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW");
File file = new File(filePath);
String fileName = file.getName();
OutputStream outputStream = connection.getOutputStream();
outputStream.write(("--\r\n").getBytes());
outputStream.write(("Content-Disposition: form-data; name=\"file\"; filename=\"" + fileName + "\"\r\n").getBytes());
outputStream.write(("Content-Type: " + HttpURLConnection.guessContentTypeFromName(fileName) + "\r\n").getBytes());
outputStream.write("\r\n".getBytes());
InputStream inputStream = FileUploader.class.getClassLoader().getResourceAsStream(filePath);
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
outputStream.write("\r\n--".getBytes());
inputStream.close();
outputStream.close();
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
System.out.println("File uploaded successfully.");
} else {
System.out.println("File upload failed with response code: " + responseCode);
}
}
public static void main(String[] args) throws IOException {
String serverUrl = "http://localhost:8080/upload";
String filePath = "example.txt";
uploadFile(serverUrl, filePath);
}
}
```
解释:
1. 创建一个URL对象来连接到文件上传服务器。
2. 创建一个HttpURLConnection对象并设置请求方法为POST。
3. 开启输出流并设置请求头为multipart/form-data,这是文件上传时必须的。
4. 创建一个文件对象,获取文件名。
5. 写入文件内容并关闭输出流。
6. 获取响应码并根据响应码输出上传结果。
注意:
1. 文件路径应相对于项目根目录或绝对路径,否则可能会出现FileNotFoundException。
2. 代码中的上传文件服务器地址应根据实际情况修改。
阅读全文
相关推荐
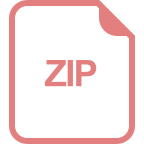
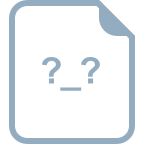
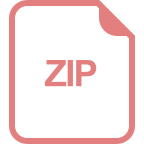
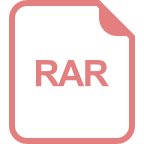
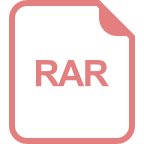
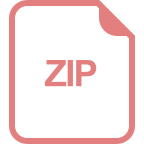
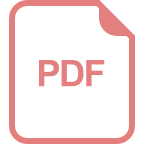
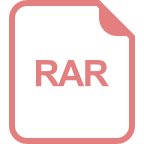
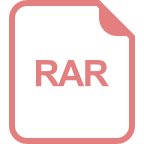
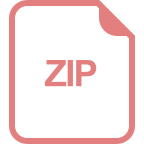
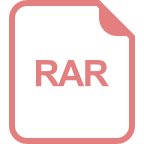
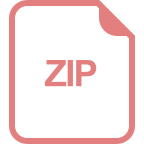
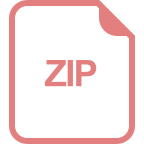