用C++写一个在linux上运行的web后台服务器
时间: 2023-11-16 15:06:48 浏览: 66
要用C++编写运行在Linux上的Web后端服务器,可以使用一些流行的网络库,例如Boost.Asio或libevent。这些库提供了处理网络请求和响应的功能,使得开发Web服务器变得更加容易。
以下是一个简单的示例程序,演示如何使用Boost.Asio来编写一个简单的Web服务器。
```cpp
#include <boost/asio.hpp>
#include <iostream>
#include <string>
using boost::asio::ip::tcp;
int main() {
try {
boost::asio::io_service io_service;
// 监听端口为8080
tcp::acceptor acceptor(io_service, tcp::endpoint(tcp::v4(), 8080));
std::cout << "Server started. Listening on port 8080." << std::endl;
while (true) {
// 等待新的连接
tcp::socket socket(io_service);
acceptor.accept(socket);
std::cout << "New connection established." << std::endl;
// 读取并解析HTTP请求
boost::asio::streambuf request_buffer;
boost::asio::read_until(socket, request_buffer, "\r\n\r\n");
std::string request_data = boost::asio::buffer_cast<const char*>(request_buffer.data());
std::cout << "Received request:\n" << request_data << std::endl;
// 构造HTTP响应
std::string response_body = "<html><body><h1>Hello, World!</h1></body></html>";
std::string response_headers =
"HTTP/1.1 200 OK\r\nContent-Length: " + std::to_string(response_body.length()) + "\r\n\r\n";
// 发送响应
boost::asio::write(socket, boost::asio::buffer(response_headers));
boost::asio::write(socket, boost::asio::buffer(response_body));
std::cout << "Response sent." << std::endl;
}
} catch (std::exception& e) {
std::cerr << "Exception: " << e.what() << std::endl;
}
return 0;
}
```
这个程序会监听8080端口,并且每当有新的连接请求时,会读取请求并发送“Hello, World!”作为响应。如果需要在更复杂的情况下使用,可以将其改写为支持多线程或异步I/O的服务器。
相关推荐
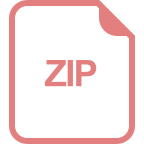
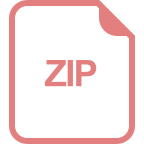
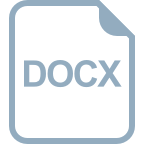














