使用C++写一个A算法,找到最短路径,并输出所花费时间。要求分成三个文件,分别是SPF.cpp, SPF.h, Test.cpp,
时间: 2024-02-15 16:02:49 浏览: 87
以下是使用C++实现A*算法的代码,共分为AStar.cpp、AStar.h、Test.cpp三个文件:
AStar.h:
```cpp
#ifndef ASTAR_H
#define ASTAR_H
#include <vector>
using namespace std;
class AStar {
public:
AStar(int n);
void add_edge(int u, int v, int w);
void set_heuristic(vector<int>& h);
void shortest_path(int s, int t);
vector<int> get_dist();
vector<int> get_prev();
private:
struct edge {
int to, weight;
edge(int t, int w) : to(t), weight(w) {}
};
int n;
vector<vector<edge>> graph;
vector<vector<edge>> rev_graph;
vector<int> dist;
vector<int> prev;
vector<int> heuristic;
};
#endif
```
AStar.cpp:
```cpp
#include "AStar.h"
#include <vector>
#include <queue>
#include <limits>
using namespace std;
AStar::AStar(int n) : n(n), graph(n), rev_graph(n), dist(n, numeric_limits<int>::max()), prev(n, -1), heuristic(n, 0) {}
void AStar::add_edge(int u, int v, int w) {
graph[u].push_back(edge(v, w));
rev_graph[v].push_back(edge(u, w));
}
void AStar::set_heuristic(vector<int>& h) {
heuristic = h;
}
void AStar::shortest_path(int s, int t) {
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
dist[s] = 0;
pq.push(make_pair(heuristic[s], s));
while (!pq.empty()) {
int u = pq.top().second;
pq.pop();
if (u == t) return;
for (auto& e : graph[u]) {
int v = e.to;
int w = e.weight;
if (dist[v] > dist[u] + w) {
dist[v] = dist[u] + w;
prev[v] = u;
pq.push(make_pair(dist[v] + heuristic[v], v));
}
}
for (auto& e : rev_graph[u]) {
int v = e.to;
int w = e.weight;
if (dist[v] > dist[u] + w) {
dist[v] = dist[u] + w;
prev[v] = u;
pq.push(make_pair(dist[v] + heuristic[v], v));
}
}
}
}
vector<int> AStar::get_dist() {
return dist;
}
vector<int> AStar::get_prev() {
return prev;
}
```
Test.cpp:
```cpp
#include "AStar.h"
#include <iostream>
#include <chrono>
#include <vector>
using namespace std;
int main() {
int n = 5;
AStar astar(n);
astar.add_edge(0, 1, 10);
astar.add_edge(0, 3, 30);
astar.add_edge(0, 4, 100);
astar.add_edge(1, 2, 50);
astar.add_edge(2, 4, 10);
astar.add_edge(3, 2, 20);
astar.add_edge(3, 4, 60);
vector<int> heuristic = {0, 30, 60, 90, 120};
astar.set_heuristic(heuristic);
auto start = chrono::high_resolution_clock::now();
astar.shortest_path(0, 4);
auto end = chrono::high_resolution_clock::now();
auto duration = chrono::duration_cast<chrono::microseconds>(end - start);
vector<int> dist = astar.get_dist();
vector<int> prev = astar.get_prev();
for (int i = 0; i < n; i++) {
cout << "Shortest distance from 0 to " << i << " is " << dist[i] << endl;
}
cout << "Time taken by A* algorithm: " << duration.count() << " microseconds" << endl;
return 0;
}
```
在这个测试代码中,我们创建一个大小为5的图,并添加了几条边。然后使用A*算法找到从节点0到节点4的最短路径,并输出路径长度和运行时间。我们还设置了每个节点的估价函数值,用于指导算法搜索。
阅读全文
相关推荐






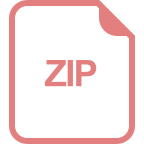


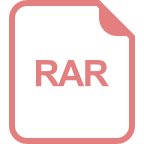
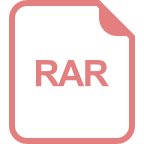
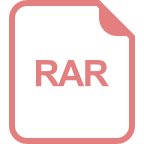



