编程完成线性表的基本操作:查找、插入和删除。编写一段程序的运行结果如下,涉及顺序表的初始化、求长度、插入删除元素、查找、输出,用C语言实现
时间: 2024-12-02 10:16:21 浏览: 16
在C语言中,我们可以使用结构体和数组来表示顺序表(数组实现),然后提供相应的函数来实现查找、插入和删除等基本操作。下面是一个简单的示例:
```c
#include <stdio.h>
// 定义顺序表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化顺序表
Node* createList() {
Node* list = (Node*)malloc(sizeof(Node));
list->data = 0;
list->next = NULL;
return list;
}
// 求列表长度
int getLength(Node* head) {
int length = 0;
Node* current = head;
while (current != NULL) {
length++;
current = current->next;
}
return length;
}
// 插入元素到列表
void insertElement(Node** head, int value, int position) {
if (position == 0) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
for (int i = 0; i < position - 1 && current != NULL; i++) {
current = current->next;
}
if (current != NULL) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = current->next;
current->next = newNode;
} else {
printf("Invalid position.\n");
}
}
}
// 删除元素
void deleteElement(Node** head, int value) {
if (*head == NULL) {
return;
}
if ((*head)->data == value) {
Node* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Node* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) {
Node* temp = current->next;
current->next = current->next->next;
free(temp);
} else {
printf("Element not found.\n");
}
}
// 查找元素并返回其索引
int findElement(Node* head, int value) {
int index = 0;
Node* current = head;
while (current != NULL) {
if (current->data == value) {
break;
}
index++;
current = current->next;
}
if (current == NULL) {
return -1; // 元素未找到
}
return index;
}
// 输出列表内容
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
int main() {
Node* myList = createList();
// 插入元素
insertElement(&myList, 5, 0); // myList: 5 -> NULL
insertElement(&myList, 10, 1); // myList: 5 -> 10 -> NULL
// 打印列表
printList(myList);
// 删除元素
deleteElement(&myList, 10); // myList: 5 -> NULL
// 查找元素
int elementIndex = findElement(myList, 5);
if (elementIndex != -1) {
printf("Found 5 at index %d.\n", elementIndex);
} else {
printf("5 not found in the list.\n");
}
// 清理内存
free(myList);
return 0;
}
```
这个程序展示了如何创建、插入、删除、查找和打印顺序表的操作。请注意,在实际应用中,应考虑错误处理和释放动态分配的内存。
阅读全文
相关推荐
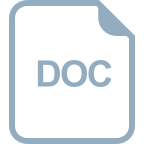
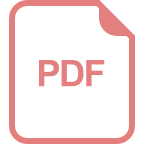
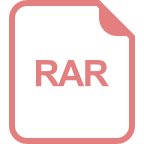

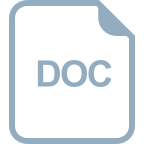
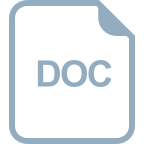
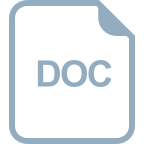
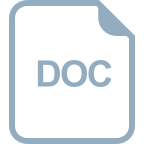
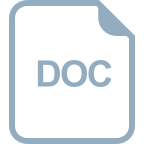
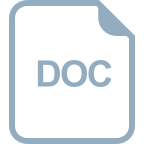
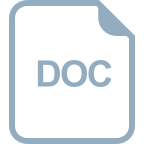
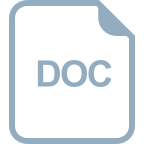
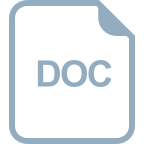
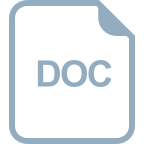
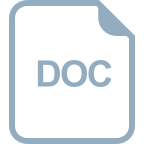
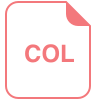
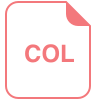
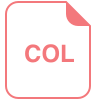
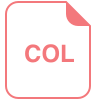