lua_newthread
时间: 2023-09-05 20:00:33 浏览: 263
lua_newthread函数是一种在Lua中创建新线程的方法。通过调用lua_newthread函数,可以创建一个新的Lua线程,并返回一个表示该线程的引用。这个引用可以用于操作和控制该线程的执行。
使用lua_newthread函数,可以在同一进程内创建多个并发执行的Lua线程。每个线程都有自己的栈空间和执行环境,可以独立执行Lua代码。这种多线程的机制可以提高编程的效率和灵活性。
在创建新线程后,可以使用lua_resume函数来启动该线程的执行,并且可以多次调用lua_resume函数来控制线程的执行流程。线程可以在不同的状态之间切换,比如从挂起状态到运行状态,再从运行状态到挂起状态等等。
通过使用lua_newthread函数,可以在Lua中实现多任务处理和并发编程。每个线程可以独立执行不同的任务,从而提高程序的并发性和响应能力。而且,由于所有线程都运行在同一进程中,它们可以共享同一份Lua状态和全局变量,方便数据的共享和通信。
总之,lua_newthread函数可以方便地在Lua中创建新的线程,实现多任务处理和并发编程。它为Lua提供了更多的编程选择和灵活性,可以满足不同程序的需求。
相关问题
lua里面写一个程序读取串口数据,用C调用lua,在c中写个线程读取同一个串口数据,要求两个读取互斥
以下是一个基本的示例程序,演示如何在 C 中创建线程读取串口数据,并使用 Lua 与该线程进行互斥。
```c
#include <stdio.h>
#include <string.h>
#include <lua.h>
#include <lauxlib.h>
#include <lualib.h>
#include <pthread.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define SERIAL_PORT "/dev/ttyUSB0"
#define BAUD_RATE B9600
int running = 1;
pthread_mutex_t mutex;
int serial_fd = -1;
void *read_serial(void *arg) {
char buffer[1024];
int bytes_read;
while (running) {
pthread_mutex_lock(&mutex);
if (serial_fd >= 0) {
bytes_read = read(serial_fd, buffer, sizeof(buffer));
if (bytes_read > 0) {
lua_State *L = (lua_State *) arg;
lua_getglobal(L, "on_serial_data");
lua_pushlstring(L, buffer, bytes_read);
lua_call(L, 1, 0);
}
}
pthread_mutex_unlock(&mutex);
usleep(1000);
}
return NULL;
}
int open_serial_port() {
int fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
fprintf(stderr, "Error opening serial port %s\n", SERIAL_PORT);
return -1;
}
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, BAUD_RATE);
cfsetospeed(&options, BAUD_RATE);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CRTSCTS;
tcsetattr(fd, TCSANOW, &options);
fcntl(fd, F_SETFL, 0);
return fd;
}
int lua_open_serial_port(lua_State *L) {
serial_fd = open_serial_port();
if (serial_fd < 0) {
lua_pushboolean(L, 0);
} else {
lua_pushboolean(L, 1);
}
return 1;
}
int lua_close_serial_port(lua_State *L) {
if (serial_fd >= 0) {
close(serial_fd);
serial_fd = -1;
}
return 0;
}
int lua_is_serial_port_open(lua_State *L) {
if (serial_fd >= 0) {
lua_pushboolean(L, 1);
} else {
lua_pushboolean(L, 0);
}
return 1;
}
int lua_start_serial_read_thread(lua_State *L) {
pthread_t thread_id;
pthread_mutex_init(&mutex, NULL);
pthread_create(&thread_id, NULL, read_serial, L);
pthread_detach(thread_id);
return 0;
}
int main(int argc, char **argv) {
lua_State *L = luaL_newstate();
luaL_openlibs(L);
lua_pushcfunction(L, lua_open_serial_port);
lua_setglobal(L, "open_serial_port");
lua_pushcfunction(L, lua_close_serial_port);
lua_setglobal(L, "close_serial_port");
lua_pushcfunction(L, lua_is_serial_port_open);
lua_setglobal(L, "is_serial_port_open");
lua_pushcfunction(L, lua_start_serial_read_thread);
lua_setglobal(L, "start_serial_read_thread");
luaL_dofile(L, "main.lua");
running = 0;
pthread_mutex_destroy(&mutex);
lua_close(L);
return 0;
}
```
在 `main.lua` 文件中,我们可以编写 Lua 代码来调用 C 函数,并在 `on_serial_data` 函数中处理串口数据。
```lua
function on_serial_data(data)
print("Received data: " .. data)
end
-- 打开串口
if not open_serial_port() then
print("Failed to open serial port")
return
end
-- 开启读取线程
start_serial_read_thread()
-- 等待用户输入退出命令
while true do
local line = io.read()
if line == "exit" then
break
end
end
-- 关闭串口
close_serial_port()
```
注意,在 `read_serial` 函数中,我们使用了一个互斥锁来保证在串口被读取时,C 和 Lua 两个线程不会同时读取串口。我们还使用了 `usleep` 函数来避免过多的 CPU 开销。
java redis lua 加锁
在Java中使用Redis和Lua脚本实现分布式锁可以确保在多个线程或多个应用程序之间同步对共享资源的访问。下面是一个示例代码:
```java
import redis.clients.jedis.Jedis;
public class RedisLock {
private static final String LOCK_KEY = "my_lock";
private static final int LOCK_EXPIRE_TIME = 10000; // 锁的过期时间,单位毫秒
private static final int ACQUIRE_TIMEOUT = 1000; // 获取锁的超时时间,单位毫秒
private Jedis jedis;
public RedisLock() {
jedis = new Jedis("localhost", 6379);
}
public boolean acquireLock() {
long start = System.currentTimeMillis();
try {
while (true) {
String result = jedis.set(LOCK_KEY, "locked", "NX", "PX", LOCK_EXPIRE_TIME);
if ("OK".equals(result)) {
return true; // 获取锁成功
}
if (System.currentTimeMillis() - start > ACQUIRE_TIMEOUT) {
return false; // 获取锁超时
}
Thread.sleep(100); // 等待重试
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
return false;
}
}
public void releaseLock() {
jedis.del(LOCK_KEY);
}
}
```
在上面的示例中,我们使用了Redis的`SET`命令来尝试获取锁。通过设置`NX`参数,可以确保只有当键不存在时才会设置成功,因此只有一个线程或应用程序能够成功获取到锁。我们还使用了`PX`参数来设置锁的过期时间,以防止死锁的情况。
如果获取锁失败或超时,可以根据实际情况进行处理,例如等待一段时间后重试或放弃获取锁。
在实际使用中,还可以结合Lua脚本来实现更复杂的锁逻辑,以满足特定的业务需求。
阅读全文
相关推荐
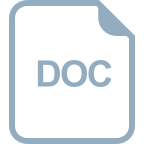
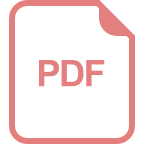
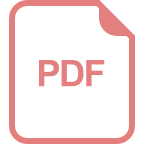













