java类的实例化数组
时间: 2024-01-04 20:17:45 浏览: 51
Java类的实例化数组是指创建一个类的多个对象,并将它们存储在一个数组中。具体实现方法如下:
1.声明一个类,例如Car类:
```java
public class Car {
private String brand;
private String color;
private int price;
public Car(String brand, String color, int price) {
this.brand = brand;
this.color = color;
this.price = price;
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
}
```
2.在另一个类中实例化Car类的数组:
```java
public class CarArray {
public static void main(String[] args) {
Car[] cars = new Car[3]; // 创建一个长度为3的Car类型数组
// 实例化Car类的对象,并将它们存储在数组中
cars[0] = new Car("BMW", "black", 500000);
cars[1] = new Car("Audi", "white", 600000);
cars[2] = new Car("Benz", "red", 700000);
// 遍历数组,输出每个Car对象的品牌、颜色和价格
for (int i = 0; i < cars.length; i++) {
System.out.println("Brand: " + cars[i].getBrand() + ", Color: " + cars[i].getColor() + ", Price: " + cars[i].getPrice());
}
}
}
```
上述代码中,我们首先创建了一个长度为3的Car类型数组,然后实例化了三个Car对象,并将它们存储在数组中。最后,我们遍历数组,输出每个Car对象的品牌、颜色和价格。
相关推荐
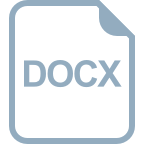














