xml悬浮窗 canvas 画布
时间: 2023-08-10 12:06:44 浏览: 53
要在XML中创建悬浮窗,你可以使用Android的WindowManager类和布局文件来实现。以下是一个示例代码:
首先,创建一个名为`floating_window.xml`的布局文件,用于定义悬浮窗的界面:
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<LinearLayout
android:id="@+id/floating_layout"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
android:background="@android:color/white"
android:elevation="8dp">
<!-- 在这里添加你想要显示的内容 -->
</LinearLayout>
</RelativeLayout>
```
在上述布局文件中,我们使用一个RelativeLayout作为根容器,并添加一个LinearLayout用于显示窗口内容。你可以在LinearLayout中添加自定义的视图或控件。
接下来,创建一个名为`FloatingWindowService.java`的服务类,用于管理悬浮窗的显示和隐藏:
```java
import android.app.Service;
import android.content.Intent;
import android.graphics.PixelFormat;
import android.os.IBinder;
import android.view.Gravity;
import android.view.LayoutInflater;
import android.view.View;
import android.view.WindowManager;
public class FloatingWindowService extends Service {
private WindowManager windowManager;
private View floatingView;
public FloatingWindowService() {
}
@Override
public IBinder onBind(Intent intent) {
return null;
}
@Override
public void onCreate() {
super.onCreate();
// 初始化WindowManager
windowManager = (WindowManager) getSystemService(WINDOW_SERVICE);
// 加载悬浮窗布局
LayoutInflater inflater = LayoutInflater.from(this);
floatingView = inflater.inflate(R.layout.floating_window, null);
// 设置悬浮窗参数
WindowManager.LayoutParams params = new WindowManager.LayoutParams(
WindowManager.LayoutParams.WRAP_CONTENT,
WindowManager.LayoutParams.WRAP_CONTENT,
WindowManager.LayoutParams.TYPE_APPLICATION_OVERLAY,
WindowManager.LayoutParams.FLAG_NOT_FOCUSABLE,
PixelFormat.TRANSLUCENT);
params.gravity = Gravity.TOP | Gravity.START;
params.x = 0;
params.y = 0;
// 将悬浮窗添加到WindowManager中
windowManager.addView(floatingView, params);
}
@Override
public void onDestroy() {
super.onDestroy();
// 移除悬浮窗
if (floatingView != null) {
windowManager.removeView(floatingView);
}
}
}
```
在上述代码中,我们创建了一个`FloatingWindowService`服务类,用于在后台管理悬浮窗的显示和隐藏。在`onCreate()`方法中,我们初始化了`WindowManager`,加载了悬浮窗布局,并设置了悬浮窗的参数。最后,将悬浮窗添加到`WindowManager`中。在`onDestroy()`方法中,我们移除了悬浮窗。
记得在`AndroidManifest.xml`文件中注册服务:
```xml
<service android:name=".FloatingWindowService"
android:enabled="true"
android:exported="true" />
```
最后,在你的活动或广播接收器中启动该服务:
```java
Intent intent = new Intent(context, FloatingWindowService.class);
context.startService(intent);
```
这样,当你启动该服务时,悬浮窗就会显示出来。你可以在`floating_window.xml`中添加你想要显示的内容,例如一个`Canvas`画布。
希望这能帮助到你!
相关推荐
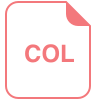













