51单片机串口接收数字在数码管显示程序用查询方法
时间: 2024-02-29 17:53:03 浏览: 125
好的,以下是一个简单的51单片机串口接收数字并在数码管上显示的程序,采用查询方法:
```c
#include <reg52.h>
#define FREQ_OSC 11059200UL
#define BAUD_RATE 9600
// 数码管引脚连接定义
sbit DIG1 = P2^0;
sbit DIG2 = P2^1;
sbit DIG3 = P2^2;
sbit DIG4 = P2^3;
sbit A = P1^0;
sbit B = P1^1;
sbit C = P1^2;
sbit D = P1^3;
sbit E = P1^4;
sbit F = P1^5;
sbit G = P1^6;
sbit DP = P1^7;
// 转换数字到数码管段码的查找表
const unsigned char digitToSegment[] = {
0x3f, // 0
0x06, // 1
0x5b, // 2
0x4f, // 3
0x66, // 4
0x6d, // 5
0x7d, // 6
0x07, // 7
0x7f, // 8
0x6f, // 9
};
// 延时函数
void delay(unsigned int ms)
{
unsigned int i, j;
for (i = 0; i < ms; i++)
for (j = 0; j < FREQ_OSC / 12000; j++);
}
// 显示一个数字到指定的位
void displayDigit(unsigned char digit, unsigned char position)
{
unsigned char segment = digitToSegment[digit];
unsigned char mask = 1 << position;
unsigned char value = 0;
// 将段码输出到数码管引脚
if (segment & 0x01) A = 1;
if (segment & 0x02) B = 1;
if (segment & 0x04) C = 1;
if (segment & 0x08) D = 1;
if (segment & 0x10) E = 1;
if (segment & 0x20) F = 1;
if (segment & 0x40) G = 1;
if (segment & 0x80) DP = 1;
// 设置要显示的位置
if (position == 0) DIG1 = 0;
if (position == 1) DIG2 = 0;
if (position == 2) DIG3 = 0;
if (position == 3) DIG4 = 0;
// 等待一段时间后关闭显示
delay(2);
DIG1 = 1;
DIG2 = 1;
DIG3 = 1;
DIG4 = 1;
// 清除数码管引脚
A = 0;
B = 0;
C = 0;
D = 0;
E = 0;
F = 0;
G = 0;
DP = 0;
}
// 主程序
void main()
{
unsigned char data;
unsigned char digits[4] = {0, 0, 0, 0};
unsigned char i;
// 初始化串口
TMOD = 0x20;
TH1 = 256 - FREQ_OSC / 32 / BAUD_RATE;
TL1 = TH1;
TR1 = 1;
SM0 = 0;
SM1 = 1;
REN = 1;
while (1) {
// 等待接收到一个数字
while (!RI);
data = SBUF;
RI = 0;
// 将数字解析为四个数字
digits[0] = data / 1000;
digits[1] = data / 100 % 10;
digits[2] = data / 10 % 10;
digits[3] = data % 10;
// 显示四个数字
for (i = 0; i < 4; i++) {
displayDigit(digits[i], i);
}
}
}
```
上述程序通过查询方法实现了串口接收数字并在数码管上显示。程序中使用了一个查找表将数字转换为数码管段码,然后使用一个循环将四个数字依次显示到数码管的四个位置上。程序中还使用了一个简单的延时函数,以等待一段时间后关闭数码管显示,避免出现闪烁的现象。
阅读全文
相关推荐
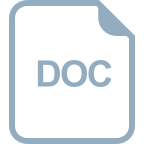
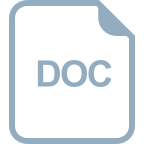
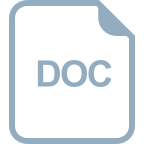

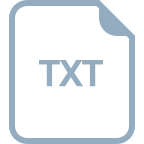
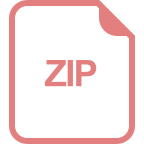
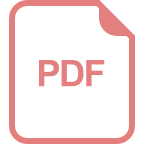
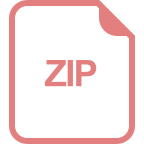
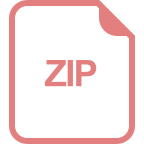
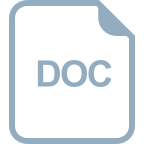






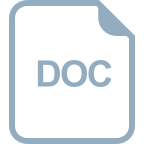