用c++写代码示例,写一个名为Robot的类,具有 int _ID, string _name, double _IQ 等 private 字段,以及在主模块的 main 函数定义一个 Robot 类型的数组并初始化,然后按 Robot 的不同字段进行排序
时间: 2024-05-19 21:15:49 浏览: 121
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
class Robot {
private:
int _ID;
string _name;
double _IQ;
public:
Robot(int ID, string name, double IQ) : _ID(ID), _name(name), _IQ(IQ) {}
// Getters
int getID() const {
return _ID;
}
string getName() const {
return _name;
}
double getIQ() const {
return _IQ;
}
// Operator overloading for sorting
bool operator < (const Robot& robot) const {
// Sort by ID
return _ID < robot._ID;
// Sort by name
// return _name < robot._name;
// Sort by IQ
// return _IQ < robot._IQ;
}
};
int main() {
// Initialize Robot array
Robot robots[] = {
Robot(2, "Zoe", 150.5),
Robot(1, "Alice", 120.3),
Robot(3, "Bob", 170.2)
};
int numRobots = sizeof(robots) / sizeof(Robot);
// Sort the robots by ID
sort(robots, robots + numRobots);
// Print the sorted robots
for (int i = 0; i < numRobots; i++) {
cout << "Robot ID: " << robots[i].getID() << endl;
cout << "Robot Name: " << robots[i].getName() << endl;
cout << "Robot IQ: " << robots[i].getIQ() << endl;
cout << endl;
}
return 0;
}
阅读全文
相关推荐
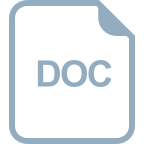
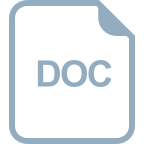
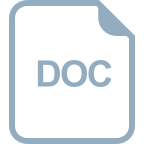
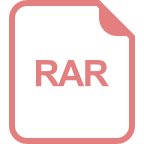
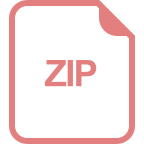
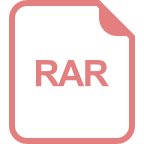
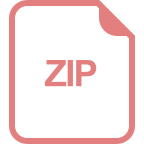
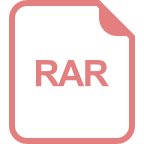
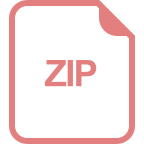
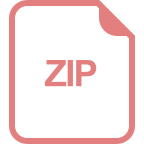
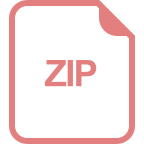
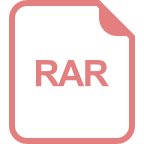
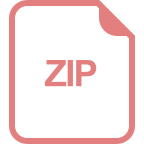
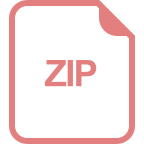