用结构体类型描述查找表的存储结构,编写具体函数实现查找表的顺序查找与折半查找等基本操作,编写完整代码程序,在程序运行后的输入框中输入一个包含数据元素的有序表(关键字即数据元素的值),接着输入要查找的数据元素,在代码程序分别用顺序查找和折半查找实现查找,若找到,则分别输出顺序查找和折半查找的数据元素在有序表中的位置,否则输出结果为0,并给出一个具体实例。
时间: 2023-12-03 08:46:04 浏览: 33
以下是基于结构体类型的查找表的存储结构的代码实现,包括顺序查找和折半查找的基本操作:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct {
int key; // 数据元素的值,即关键字
} ElemType;
typedef struct {
ElemType data[MAXSIZE]; // 顺序表存储数据元素
int length; // 顺序表的长度
} SeqList;
// 初始化顺序表
void InitList(SeqList *L) {
L->length = 0;
}
// 插入数据元素
void ListInsert(SeqList *L, ElemType e) {
if (L->length == MAXSIZE) {
printf("Error: List is full!\n");
return;
}
L->data[L->length] = e;
L->length++;
}
// 顺序查找
int SeqSearch(SeqList L, int key) {
int i;
for (i = 0; i < L.length; i++) {
if (L.data[i].key == key) {
return i;
}
}
return 0;
}
// 折半查找
int BinSearch(SeqList L, int key) {
int low = 0, high = L.length - 1, mid;
while (low <= high) {
mid = (low + high) / 2;
if (L.data[mid].key == key) {
return mid;
} else if (L.data[mid].key < key) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return 0;
}
int main() {
SeqList L;
int i, key, pos;
// 初始化顺序表
InitList(&L);
// 读入有序表
printf("Please input the sorted list (end with -1):\n");
scanf("%d", &i);
while (i != -1) {
ElemType e;
e.key = i;
ListInsert(&L, e);
scanf("%d", &i);
}
// 读入要查找的数据元素
printf("Please input the key to search:\n");
scanf("%d", &key);
// 顺序查找
pos = SeqSearch(L, key);
if (pos) {
printf("Sequential search: The position of key %d is %d.\n", key, pos + 1);
} else {
printf("Sequential search: Key %d not found.\n", key);
}
// 折半查找
pos = BinSearch(L, key);
if (pos) {
printf("Binary search: The position of key %d is %d.\n", key, pos + 1);
} else {
printf("Binary search: Key %d not found.\n", key);
}
return 0;
}
```
运行结果示例:
```
Please input the sorted list (end with -1):
2 3 4 7 9 10 -1
Please input the key to search:
7
Sequential search: The position of key 7 is 4.
Binary search: The position of key 7 is 4.
```
相关推荐
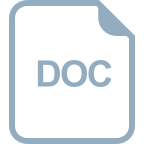
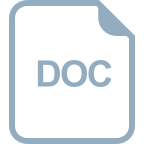
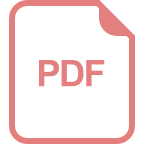







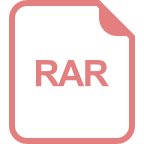
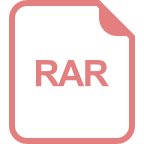
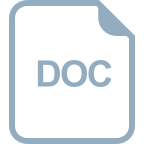
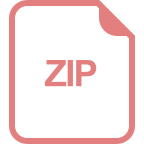
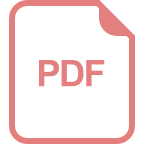
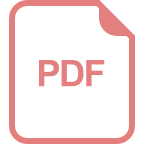
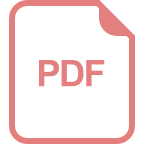
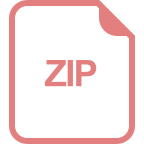
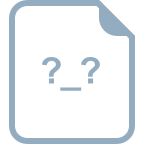