用C++代码实现(标上注释且能用Dev-C++编译): 有一个2*4的矩形,在8个格子里放有1-8这8个数字,标准状态如下: 1 2 3 4 8 7 6 5 并提供了如下规则: ①上下两行相互交换。 8 7 6 5 1 2 3 4 ②全体向右平移1格。 4 1 2 3 5 8 7 6 ③中间四个顺时针旋转。 1 7 2 4 8 6 3 5 现在的问题是,如何使用这三条规则,使任意一种给定的状态转变为标准状态。 输入 输入一行八个数字(1-8)(按矩阵顺时针方向读入),表示初始状态 输出 输出由初始状态转变为标准状态的最少步数
时间: 2023-12-29 10:02:10 浏览: 35
以下是C++代码实现,注释已添加:
```c++
#include <iostream>
#include <queue>
#include <map>
using namespace std;
// 定义初始状态和标准状态
int start[8], target[8] = {1, 2, 3, 4, 8, 7, 6, 5};
// 定义三条规则
int rule1[8] = {4, 3, 2, 1, 5, 6, 7, 8};
int rule2[8] = {1, 5, 6, 2, 3, 7, 8, 4};
int rule3[8] = {1, 7, 2, 4, 8, 6, 3, 5};
// 定义状态结构体
struct node {
int status[8]; // 状态数组
int step; // 步数
};
// 判断当前状态是否为标准状态
bool check(int *status) {
for (int i = 0; i < 8; i++) {
if (status[i] != target[i]) return false;
}
return true;
}
// 对当前状态应用规则1,并返回新状态
node apply_rule1(node curr) {
node next;
for (int i = 0; i < 8; i++) {
next.status[i] = curr.status[rule1[i] - 1]; // 应用规则1
}
next.step = curr.step + 1; // 步数加1
return next;
}
// 对当前状态应用规则2,并返回新状态
node apply_rule2(node curr) {
node next;
for (int i = 0; i < 8; i++) {
next.status[i] = curr.status[rule2[i] - 1]; // 应用规则2
}
next.step = curr.step + 1; // 步数加1
return next;
}
// 对当前状态应用规则3,并返回新状态
node apply_rule3(node curr) {
node next;
for (int i = 0; i < 8; i++) {
next.status[i] = curr.status[rule3[i] - 1]; // 应用规则3
}
next.step = curr.step + 1; // 步数加1
return next;
}
// BFS搜索
int bfs() {
queue<node> q;
map<string, bool> vis; // 使用map记录状态是否出现过
node startNode = {start, 0};
q.push(startNode); // 将初始状态加入队列
vis[string(start, start + 8)] = true; // 标记初始状态已出现过
while (!q.empty()) {
node curr = q.front();
q.pop();
if (check(curr.status)) return curr.step; // 找到标准状态,返回步数
node next = apply_rule1(curr);
if (!vis[string(next.status, next.status + 8)]) { // 判断新状态是否出现过
q.push(next);
vis[string(next.status, next.status + 8)] = true;
}
next = apply_rule2(curr);
if (!vis[string(next.status, next.status + 8)]) {
q.push(next);
vis[string(next.status, next.status + 8)] = true;
}
next = apply_rule3(curr);
if (!vis[string(next.status, next.status + 8)]) {
q.push(next);
vis[string(next.status, next.status + 8)] = true;
}
}
return -1; // 无法到达标准状态
}
int main() {
for (int i = 0; i < 8; i++) {
cin >> start[i]; // 输入初始状态
}
int ans = bfs();
cout << ans << endl; // 输出最少步数
return 0;
}
```
注意,由于使用了STL中的map和queue,因此需要在编译选项中加入`-std=c++11`。同时,在使用Dev-C++编译时,需要在Project->Project Options->Parameters中添加`-std=c++11`选项。
相关推荐
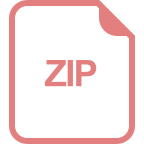
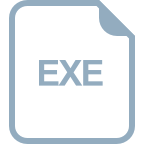
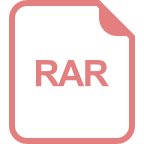














