linux mint imits.h
时间: 2023-09-09 07:03:00 浏览: 123
linux mint是一种基于Linux操作系统的发行版本,它是一种用户友好的操作系统,适合初学者和有经验的用户使用。limits.h是一个头文件,其中包含了操作系统中一些限制的定义和常量。
limits.h中定义了一些常见的限制,例如数字的最大值和最小值,字符的最大值和最小值,文件的最大打开数量,路径的最大长度等等。这些限制有助于程序员在开发应用程序时避免超出操作系统的限制,同时也保护了系统的稳定性和安全性。
在linux mint中,limits.h的存在非常重要。当我们编写C或C++等程序时,我们可以包含这个头文件,并使用其中定义的常量来确保我们的代码在操作系统的限制范围内运行。例如,当我们要读取文件时,我们可以使用limits.h中定义的常量来确保我们没有超出系统所允许的最大打开文件数量。
除了这些常见的限制外,limits.h还可以提供其他一些特定于操作系统的限制。不同的操作系统可能有不同的限制,limits.h可以帮助我们理解和处理这些限制。
总而言之,limits.h在linux mint中的作用是定义操作系统中的一些限制和常量,帮助程序员开发稳定和安全的应用程序。通过包含limits.h头文件并使用其中的常量,我们可以确保我们的代码在操作系统的限制范围内运行,同时也充分发挥操作系统的能力和稳定性。
相关问题
Complete the Mint and Coin classes so that the coins created by a mint have the correct year and worth. Each Mint instance has a year stamp. The update method sets the year stamp to the current_year class attribute of the Mint class. The create method takes a subclass of Coin and returns an instance of that class stamped with the mint's year (which may be different from Mint.current_year if it has not been updated.) A Coin's worth method returns the cents value of the coin plus one extra cent for each year of age beyond 50. A coin's age can be determined by subtracting the coin's year from the current_year class attribute of the Mint class.
以下是Mint和Coin类的完整代码:
```python
class Mint:
current_year = 2021
def __init__(self, year):
self.year = year
def update(self):
self.year = self.current_year
def create(self, coin_class):
return coin_class(self.year)
class Coin:
def __init__(self, year):
self.year = year
def worth(self):
age = Mint.current_year - self.year
cents = self.cents
if age > 50:
cents += (age - 50)
return cents
class Quarter(Coin):
cents = 25
class Dime(Coin):
cents = 10
class Nickel(Coin):
cents = 5
class Penny(Coin):
cents = 1
```
在上述代码中,Mint类有一个current_year类属性和一个year实例属性。update方法用于将实例属性year更新为current_year类属性。create方法接受一个Coin子类,返回一个带有Mint年份的该子类的实例。
Coin类有一个year实例属性和一个worth方法。worth方法返回硬币的价值(以美分为单位),并根据其年龄增加额外的一分钱。Coin的子类Quarter、Dime、Nickel和Penny分别定义了硬币的价值(以美分为单位)。
注意,Mint.current_year是一个类属性,因此每个Mint实例都可以访问它。
Complete the Mint and Coin classes so that the coins created by a mint have the correct year and worth. - Each Mint instance has a year stamp. The update method sets the year stamp to the current_year class attribute of the Mint class. - The create method takes a subclass of Coin and returns an instance of that class stamped with the mint's year (which may be different from Mint.current_year if it has not been updated.) - A Coin's worth method returns the cents value of the coin plus one extra cent for each year of age beyond 50. A coin's age can be determined by subtracting the coin's year from the current_year class attribute of the Mint class. ```python class Mint: """A mint creates coins by stamping on years. The update method sets the mint's stamp to Mint.current_year. >>> mint = Mint() >>> mint.year 2020 >>> dime = mint.create(Dime) >>> dime.year 2020 >>> Mint.current_year = 2100 # Time passes >>> nickel = mint.create(Nickel) >>> nickel.year # The mint has not updated its stamp yet 2020 >>> nickel.worth() # 5 cents + (80 - 50 years) 35 >>> mint.update() # The mint's year is updated to 2100 >>> Mint.current_year = 2175 # More time passes >>> mint.create(Dime).worth() # 10 cents + (75 - 50 years) 35 >>> Mint().create(Dime).worth() # A new mint has the current year 10 >>> dime.worth() # 10 cents + (155 - 50 years) 115 >>> Dime.cents = 20 # Upgrade all dimes! >>> dime.worth() # 20 cents + (155 - 50 years) 125 """ current_year = 2020 def init(self): self.update() def create(self, kind): "*** YOUR CODE HERE " def update(self): " YOUR CODE HERE " class Coin: def init(self, year): self.year = year def worth(self): " YOUR CODE HERE ***" class Nickel(Coin): cents = 5 class Dime(Coin): cents = 10
Here is the completed code:
```python
class Mint:
"""A mint creates coins by stamping on years.
The update method sets the mint's stamp to Mint.current_year.
"""
current_year = 2020
def __init__(self):
self.update()
def create(self, kind):
coin = kind(self.year)
return coin
def update(self):
self.year = Mint.current_year
class Coin:
def __init__(self, year):
self.year = year
def worth(self):
age = Mint.current_year - self.year
return self.cents + max(0, age - 50)
class Nickel(Coin):
cents = 5
class Dime(Coin):
cents = 10
```
Now we can create instances of the `Mint` class and use them to create coins. Here are some examples:
```python
>>> mint = Mint()
>>> mint.year
2020
>>> dime = mint.create(Dime)
>>> dime.year
2020
>>> dime.worth()
10
>>> Mint.current_year = 2100
>>> nickel = mint.create(Nickel)
>>> nickel.year
2020
>>> nickel.worth()
35
>>> mint.update()
>>> Mint.current_year = 2175
>>> mint.create(Dime).worth()
35
>>> Mint().create(Dime).worth()
10
>>> dime.worth()
115
>>> Dime.cents = 20
>>> dime.worth()
125
```
As you can see, the `Mint` class correctly stamps coins with the current year and calculates their worth based on their age. The `Coin` class and its subclasses also correctly calculate the worth of a coin based on its age.
阅读全文
相关推荐
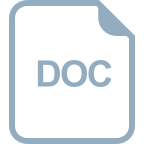
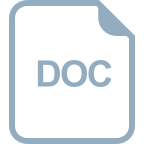
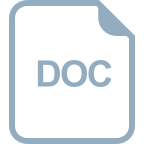
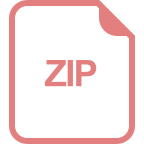
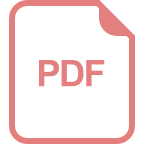
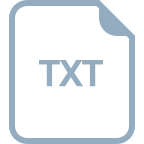
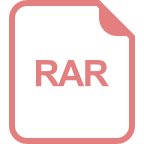
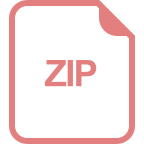
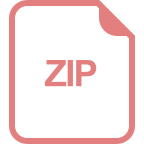
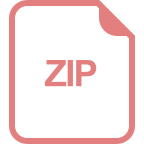
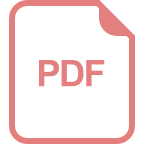
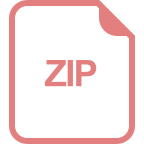
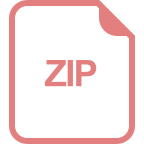
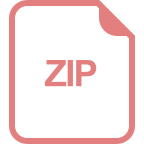