使用c#实现 验证同一个excel表中两个sheet中的一列数据去重后是否相符然后做颜色提示
时间: 2024-03-27 17:40:10 浏览: 16
可以使用C#程序来实现验证同一个Excel表中两个sheet中的一列数据去重后是否相符,并做颜色提示。具体步骤如下:
1. 引用Excel Interop库,打开Excel文件,读取需要比较的列数据,存储到List中。
```
using Excel = Microsoft.Office.Interop.Excel;
// 打开Excel文件
Excel.Application excel = new Excel.Application();
Excel.Workbook workbook = excel.Workbooks.Open(@"C:\test.xlsx");
// 读取第一个sheet中需要比较的列数据
Excel.Worksheet sheet1 = (Excel.Worksheet)workbook.Worksheets.get_Item(1);
Excel.Range range1 = sheet1.Range[sheet1.Cells[1, 1], sheet1.Cells[sheet1.UsedRange.Rows.Count, 1]];
List<string> list1 = new List<string>();
foreach (Excel.Range cell in range1.Cells)
{
string value = cell.Value.ToString();
if (!string.IsNullOrEmpty(value))
{
list1.Add(value);
}
}
// 读取第二个sheet中需要比较的列数据
Excel.Worksheet sheet2 = (Excel.Worksheet)workbook.Worksheets.get_Item(2);
Excel.Range range2 = sheet2.Range[sheet2.Cells[1, 1], sheet2.Cells[sheet2.UsedRange.Rows.Count, 1]];
List<string> list2 = new List<string>();
foreach (Excel.Range cell in range2.Cells)
{
string value = cell.Value.ToString();
if (!string.IsNullOrEmpty(value))
{
list2.Add(value);
}
}
// 关闭Excel文件
workbook.Close(false, Type.Missing, Type.Missing);
excel.Quit();
```
2. 对两个List进行去重操作。
```
// 对两个List进行去重操作
List<string> distinctList1 = list1.Distinct().ToList();
List<string> distinctList2 = list2.Distinct().ToList();
```
3. 比较两个去重后的List是否相等,如果相等则在两个sheet中对应的单元格上添加颜色。
```
// 比较两个去重后的List是否相等,如果相等则在两个sheet中对应的单元格上添加颜色
if (distinctList1.SequenceEqual(distinctList2))
{
// 设置第一个sheet中需要比较的列的颜色
Excel.Range rangeFormat1 = sheet1.Range[sheet1.Cells[1, 1], sheet1.Cells[sheet1.UsedRange.Rows.Count, 1]];
foreach (Excel.Range cell in rangeFormat1.Cells)
{
string value = cell.Value.ToString();
if (!string.IsNullOrEmpty(value))
{
if (distinctList1.Contains(value))
{
cell.Interior.Color = System.Drawing.ColorTranslator.ToOle(System.Drawing.Color.Red);
}
}
}
// 设置第二个sheet中需要比较的列的颜色
Excel.Range rangeFormat2 = sheet2.Range[sheet2.Cells[1, 1], sheet2.Cells[sheet2.UsedRange.Rows.Count, 1]];
foreach (Excel.Range cell in rangeFormat2.Cells)
{
string value = cell.Value.ToString();
if (!string.IsNullOrEmpty(value))
{
if (distinctList1.Contains(value))
{
cell.Interior.Color = System.Drawing.ColorTranslator.ToOle(System.Drawing.Color.Red);
}
}
}
}
```
注意,以上代码中的Excel文件路径和需要比较的列数可以根据具体情况进行修改。
相关推荐
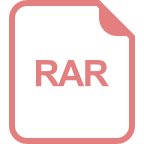
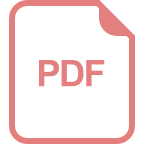














