springboot写个redis定时任务上传插入语句集合到数据库
时间: 2024-01-18 14:04:01 浏览: 186
首先,在pom.xml文件中添加Redis和MySQL的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
```
接着,在application.properties文件中添加Redis和MySQL的配置:
```properties
# Redis配置
spring.redis.host=127.0.0.1
spring.redis.port=6379
spring.redis.password=
# 数据库配置
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf8&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
```
然后,创建一个定时任务类,实现将Redis中的数据上传到MySQL的功能:
```java
@Component
public class RedisUploadTask {
private static final String REDIS_KEY = "MY_KEY"; // Redis中存储数据的键名
private static final long PERIOD = 30 * 60 * 1000; // 定时任务执行的周期,这里设置为30分钟
@Autowired
private StringRedisTemplate redisTemplate;
@Autowired
private JdbcTemplate jdbcTemplate;
@Scheduled(fixedDelay = PERIOD)
public void uploadRedisDataToMysql() {
Set<String> dataSet = redisTemplate.opsForSet().members(REDIS_KEY); // 获取Redis中的数据集合
List<String> sqlList = new ArrayList<>();
for (String data : dataSet) {
String sql = "INSERT INTO my_table (data) VALUES ('" + data + "')"; // 拼接插入语句
sqlList.add(sql);
}
jdbcTemplate.batchUpdate(sqlList.toArray(new String[sqlList.size()])); // 批量执行插入语句
}
}
```
在上面的代码中,我们使用了Spring Boot提供的StringRedisTemplate和JdbcTemplate来分别访问Redis和MySQL数据库。在定时任务方法中,我们首先通过redisTemplate获取Redis中存储的数据集合,然后遍历集合,将每个元素拼接成插入语句,并将这些语句添加到一个List中。最后,我们使用jdbcTemplate的batchUpdate方法批量执行这些插入语句,将数据上传到MySQL数据库中。
最后,我们需要在启动类上加上@EnableScheduling注解,启用定时任务功能:
```java
@SpringBootApplication
@EnableScheduling
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
这样,当我们每隔30分钟就会执行一次RedisUploadTask中的uploadRedisDataToMysql方法,将Redis中的数据集合上传到MySQL中。
阅读全文
相关推荐

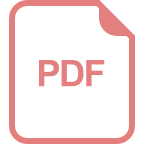
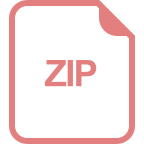
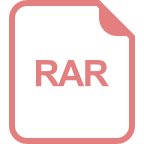
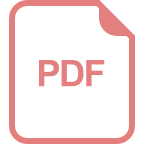
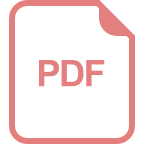
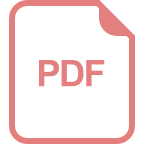
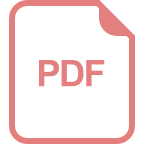
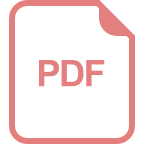
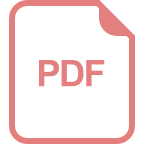
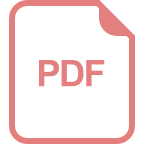
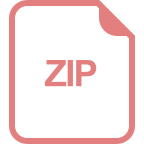
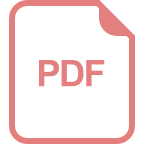
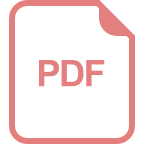
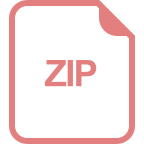