python 三维点云边界提取
时间: 2023-09-23 15:10:48 浏览: 180
您好!感谢您对ChitGPT的信任。关于您的问题,我可以为您提供一些参考信息。
要从三维点云中提取边界,您可以考虑使用几何计算或机器学习方法,具体取决于您的应用场景和数据。以下是一些可能有用的工具和技术:
1. 几何计算方法:例如,您可以使用Delaunay三角剖分、渐进凸包或Alpha形状等算法来计算点云的表面与周围环境的几何形状。然后可以使用一些空间分割算法(如RANSAC、Hough变换等)来找到边界。此外,还可以利用曲率、法线方向等几何特征来检测边界。
2. 机器学习方法:您可以使用强化学习、深度学习等机器学习算法,通过训练模型来自动识别点云边界。例如,可以使用卷积神经网络(CNN)、递归神经网络(RNN)等模型,可以使用点云分类、点云分割等任务来训练模型。
希望这些信息能帮助到您。如果您有任何其他问题,请随时问我。
相关问题
基于python的点云边缘提取算法
基于Python的点云边缘提取算法是一种用于从点云数据中提取边缘信息的方法。点云是由大量的点组成的三维坐标数据集,边缘则是点云中物体之间或物体与背景之间的边界。
基于Python的点云边缘提取算法可以分为以下几个步骤:
1. 数据预处理:从点云数据集中读取数据,并对数据进行坐标转换、滤波等预处理操作,以提高后续操作的效果。
2. 特征提取:根据特定的算法将点云中的边缘特征提取出来。常用的边缘提取算法包括基于梯度的方法、基于曲率的方法等。例如,可以计算每个点的曲率,较高的曲率值可能表示该点处的边缘。
3. 边缘点筛选:根据提取的特征,对点云中的边缘点进行筛选。可以使用阈值或其他规则来确定哪些点属于边缘。
4. 边缘重建:对筛选后的边缘点进行重建,可以使用曲线拟合等方法将离散的点连接成连续的边缘线。
5. 可视化:将提取到的边缘线以可视化的方式呈现,可以使用Python中的可视化库(如Matplotlib)进行展示,以便进一步分析和应用。
基于Python的点云边缘提取算法可以应用于各种领域,如机器人导航、三维重建等。它可以帮助我们从点云数据中提取出有用的边缘信息,从而更好地理解物体的形状和结构。同时,Python作为一种简洁易读的编程语言,为算法的实现提供了便利。
使用python,写出alpha shape算法对二维点云进行边界提取
好的,下面是使用Python实现alpha shape算法对二维点云进行边界提取的代码:
```python
from scipy.spatial import Delaunay
import numpy as np
from matplotlib import pyplot as plt
def alpha_shape(points, alpha):
"""
Compute the alpha shape (concave hull) of a set of points.
"""
# Delaunay triangulation
tri = Delaunay(points)
# Filter triangles by alpha value
edges = set()
edge_points = []
for j, tri in enumerate(tri.simplices):
# Calculate circumcenter and radius of circumcircle
x, y = np.mean(points[tri], axis=0)
r = np.sqrt((points[tri[0]][0] - x)**2 + (points[tri[0]][1] - y)**2)
# Check if triangle is within alpha value
if r < alpha:
for i in range(3):
edge = (tri[i], tri[(i+1)%3])
if edge not in edges and (edge[1], edge[0]) not in edges:
edges.add(edge)
edge_points.append(points[tri[i]])
edge_points.append(points[tri[(i+1)%3]])
# Compute boundary vertices and edges
boundary_vertices = set()
boundary_edges = []
for i, point in enumerate(points):
for edge in edges:
if i in edge:
boundary_vertices.add(i)
boundary_edges.append(edge)
# Plot alpha shape
plt.triplot(points[:,0], points[:,1], tri.simplices.copy())
plt.plot(edge_points[:,0], edge_points[:,1], 'r.')
plt.plot(points[list(boundary_vertices),0], points[list(boundary_vertices),1], 'b.')
plt.show()
return boundary_vertices, boundary_edges
```
在这个代码中,我们使用了`scipy.spatial`库中的`Delaunay`函数来进行Delaunay三角剖分,然后根据alpha值筛选出在范围内的三角形,并提取出边界。
下面是一个使用示例:
```python
# Generate random points
points = np.random.rand(30, 2)
# Compute alpha shape
alpha = 0.3
boundary_vertices, boundary_edges = alpha_shape(points, alpha)
# Print boundary vertices and edges
print("Boundary vertices:", boundary_vertices)
print("Boundary edges:", boundary_edges)
```
这个例子中,我们先生成了30个随机点,然后使用`alpha_shape`函数计算alpha shape,并输出边界点和边。
阅读全文
相关推荐
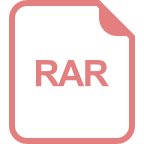
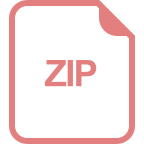
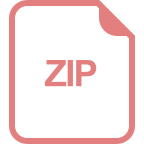











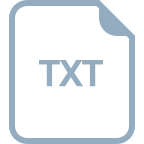
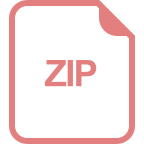