网购订单模拟 ▪ 顾客类(属性/字段:姓名、地址、订单列表(数组);方法:1.计算所有订单的总价、 总重量、和总运费,2.打印总价和运费) ▪ 订单类(属性/字段:购买商品列表(数组);方法:计算订单总价,计算总
时间: 2023-06-19 14:09:07 浏览: 70
重量,计算总运费) ▪ 商品类(属性/字段:名称、价格、重量)
以下是代码示例:
```python
class Product:
def __init__(self, name, price, weight):
self.name = name
self.price = price
self.weight = weight
class Order:
def __init__(self, products):
self.products = products
def calculate_total_price(self):
total_price = 0
for product in self.products:
total_price += product.price
return total_price
def calculate_total_weight(self):
total_weight = 0
for product in self.products:
total_weight += product.weight
return total_weight
def calculate_total_shipping_cost(self):
total_weight = self.calculate_total_weight()
total_shipping_cost = 0
if total_weight <= 10:
total_shipping_cost = 5
elif total_weight > 10 and total_weight <= 20:
total_shipping_cost = 10
elif total_weight > 20 and total_weight <= 30:
total_shipping_cost = 15
else:
total_shipping_cost = 20
return total_shipping_cost
class Customer:
def __init__(self, name, address, orders):
self.name = name
self.address = address
self.orders = orders
def calculate_total_price(self):
total_price = 0
for order in self.orders:
total_price += order.calculate_total_price()
return total_price
def calculate_total_weight(self):
total_weight = 0
for order in self.orders:
total_weight += order.calculate_total_weight()
return total_weight
def calculate_total_shipping_cost(self):
total_shipping_cost = 0
for order in self.orders:
total_shipping_cost += order.calculate_total_shipping_cost()
return total_shipping_cost
def print_total_price_and_shipping_cost(self):
total_price = self.calculate_total_price()
total_shipping_cost = self.calculate_total_shipping_cost()
print("Total price: ${}".format(total_price))
print("Total shipping cost: ${}".format(total_shipping_cost))
```
使用示例:
```python
product1 = Product("iPhone", 999, 0.5)
product2 = Product("MacBook", 1499, 1.5)
product3 = Product("iPad", 599, 0.8)
order1 = Order([product1, product2])
order2 = Order([product3])
customer = Customer("John", "123 Main St, Anytown, USA", [order1, order2])
customer.print_total_price_and_shipping_cost()
```
输出结果:
```
Total price: $2498
Total shipping cost: $15
```
阅读全文
相关推荐
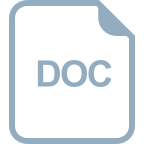
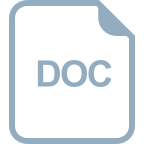
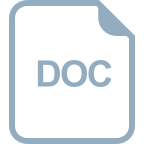
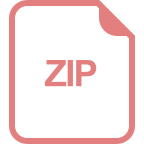
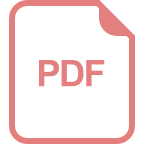
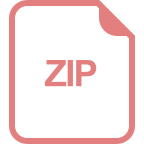
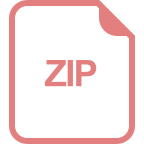
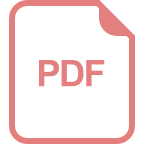
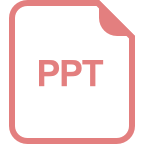
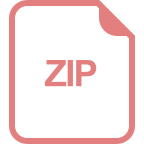
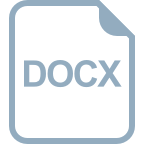
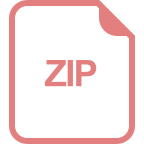
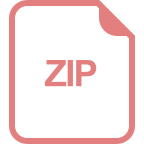
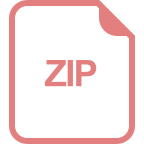
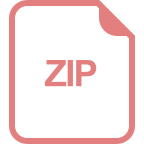
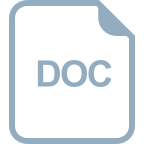
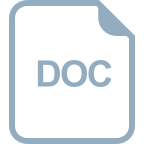
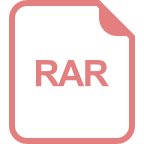
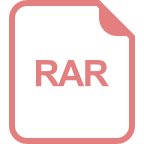