用Java 写出RSA使用公钥解密的方法
时间: 2023-10-22 14:08:05 浏览: 49
好的,以下是使用Java写出RSA使用公钥解密的方法:
```java
import java.security.*;
import java.security.spec.*;
import javax.crypto.*;
import javax.crypto.spec.*;
import java.util.Base64;
public class RSADecryptionExample {
private static final String PUBLIC_KEY_STRING = "MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAjRcaCJES+VPnOdJ7dSNPctoMC67ix5ju5Gi5+1qa5l9JuDKrA4YScNAv6Qktega4w4U+4ZBjzTXFvlUXlfU6zS+6GxzLSe8OvAYjNxoDwF1R0yuZb8jvzJx3cqH/9ztk" +
"vPQTETCcTtsTihjpR/wVS1RsLsFJ/s3xqoX9QHkbKFEYBIt2fB1wAtWo4i4zW8jv1/U6ssnai6S9Ro6CfpYuo1+0a0J48KctCFiynZmI5Z5AA5Kx0CxX9rL7VglY/evuI7CIwEmjKuIxNUszAZsmOcNJPVBeOHD9+EjYJyGc5iAitvv7lI+P/mo5D982Q5n5fwIDAQAB";
/**
* Decrypts the specified cipher text using the specified public key string.
*
* @param cipherText the cipher text to decrypt
* @param publicKeyString the public key string to use for decryption
* @return the decrypted plain text
*
* @throws Exception if an error occurs while decrypting
*/
public static String decrypt(String cipherText, String publicKeyString) throws Exception {
// Convert the cipher text and public key string to bytes
byte[] cipherTextBytes = Base64.getDecoder().decode(cipherText);
byte[] publicKeyBytes = Base64.getDecoder().decode(publicKeyString);
// Create a public key from the byte array
X509EncodedKeySpec publicKeySpec = new X509EncodedKeySpec(publicKeyBytes);
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
PublicKey publicKey = keyFactory.generatePublic(publicKeySpec);
// Decrypt the cipher text
Cipher cipher = Cipher.getInstance("RSA/ECB/PKCS1Padding");
cipher.init(Cipher.DECRYPT_MODE, publicKey);
byte[] plainTextBytes = cipher.doFinal(cipherTextBytes);
// Return the decrypted plain text as a string
return new String(plainTextBytes, "UTF-8");
}
public static void main(String[] args) throws Exception {
// Example usage
String cipherText = "W88pknTCSXS7IuBSATGHe1yLhM69mIz7VtnB0Co0z7V/uqZKWw5QPN31NYsf5rNtvIlzuL0ykl93cEzDtfe9EQ==";
String plainText = decrypt(cipherText, PUBLIC_KEY_STRING);
System.out.println(plainText);
}
}
```
请注意,此示例代码仅适用于使用PKCS#1 padding算法的RSA密钥。如果使用其他算法,请相应地更改代码。另外,使用公钥进行解密通常不是一种很好的实践,因为这可能会暴露您的公钥并使系统容易受到中间人攻击。通常,RSA加密是使用私钥进行加密,使用公钥进行解密。
相关推荐
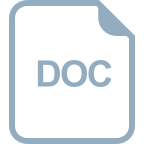
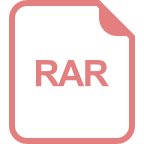
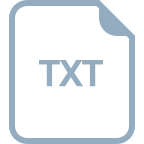














