cpp argv字符串转int
时间: 2023-08-20 12:09:18 浏览: 101
你可以使用C++的标准库函数`std::stoi`将`argv`字符串转换为整数。`argv`是一个字符串数组,其中存储了命令行参数。下面是一个示例代码:
```cpp
#include <iostream>
#include <string>
int main(int argc, char* argv[]) {
if (argc < 2) {
std::cout << "请输入一个整数作为命令行参数!" << std::endl;
return 1;
}
std::string arg = argv[1];
int num = std::stoi(arg);
std::cout << "转换后的整数是:" << num << std::endl;
return 0;
}
```
在上面的示例中,我们首先检查命令行参数的数量,确保至少有一个参数传入。然后,我们将第一个参数(`argv[1]`)作为字符串存储在`arg`中,然后使用`std::stoi`将其转换为整数存储在`num`中。最后,我们将转换后的整数输出到控制台。
请注意,如果无法成功转换为整数,`std::stoi`会抛出一个`std::invalid_argument`或`std::out_of_range`异常。因此,你可以使用适当的异常处理来处理这些异常情况。
相关问题
编写c++程序,用二进制方式打开指定的一个文件,在每一行前加行号.编写程序 lab11_2.cpp 使用 int main(int argc,char * argv[])函数中的参数传递操作的文件名,声明 ofstream 的对象对文件进行操作,使用 getline 成员函数读入数据,使用cout 输出字符到文件。
以下是lab11_2.cpp程序的代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main(int argc, char *argv[]) {
if (argc < 2) {
cout << "Usage: " << argv[0] << " FILENAME" << endl;
return 1;
}
ifstream fin(argv[1], ios::binary);
if (!fin) {
cout << "Failed to open file: " << argv[1] << endl;
return 1;
}
ofstream fout("output.txt", ios::binary);
if (!fout) {
cout << "Failed to create output file" << endl;
return 1;
}
string line;
int line_number = 1;
while (getline(fin, line)) {
fout << line_number++ << " " << line << endl;
}
fin.close();
fout.close();
cout << "Done! Check out the file 'output.txt'." << endl;
return 0;
}
```
这个程序会打开一个二进制文件,然后在每一行前加上行号,并输出到一个名为output.txt的新文件中。你可以将你想要操作的文件名作为参数传递给程序来操作它,例如:
```
./lab11_2.exe input.txt
```
这将会将input.txt的内容加上行号并输出到output.txt中。
#include <iostream> #include <fstream> #include <vector> #include <unordered_map> using namespace std; void compress(const string& inputFilePath, const string& outputFilePath) { // 读取输入文件 ifstream inputFile(inputFilePath, ios::binary); // 构建字典 unordered_map<string, int> dictionary; int dictionarySize = 256; for (int i = 0; i < 256; i++) { dictionary[string(1, i)] = i; } vector<int> compressedData; string currentString; char inputChar; while (inputFile.get(inputChar)) { // 获取当前字符串 string newString = currentString + inputChar; // 当前字符串在字典中存在时继续添加字符 if (dictionary.find(newString) != dictionary.end()) { currentString = newString; } // 当前字符串不在字典中时 else { // 将当前字符串添加到压缩数据中 compressedData.push_back(dictionary[currentString]); // 将新的字符串添加到字典中 dictionary[newString] = dictionarySize++; currentString = string(1, inputChar); } } // 处理最后一组字符串 if (!currentString.empty()) { compressedData.push_back(dictionary[currentString]); } // 输出到文件 ofstream outputFile(outputFilePath, ios::binary); for (const int& code : compressedData) { outputFile.write((char*)&code, sizeof(int)); } } int main(int argc, char* argv[]) { if (argc < 3) { cout << "Usage: LZWCompressor <input_file> <output_file>" << endl; } else { string inputFilePath = argv[1]; string outputFilePath = argv[2]; compress(inputFilePath, outputFilePath); } return 0; }如何使用这段代码压缩文件
你可以按照以下步骤使用这段代码压缩文件:
1. 将代码保存为LZWCompressor.cpp文件。
2. 在命令行中使用以下命令编译代码:
```g++ LZWCompressor.cpp -o LZWCompressor```
3. 在命令行中使用以下命令运行程序并压缩文件:
```./LZWCompressor input_file output_file```
其中,input_file为待压缩的文件路径,output_file为压缩后的文件路径。
4. 程序运行结束后,将生成一个压缩后的文件,文件名为output_file。
阅读全文
相关推荐
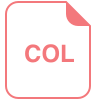











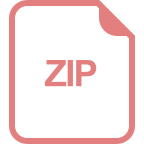
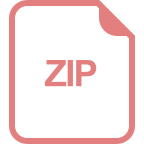
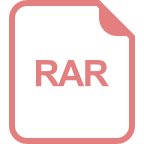
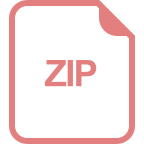