python爬取天气可视化
时间: 2023-09-22 20:14:04 浏览: 102
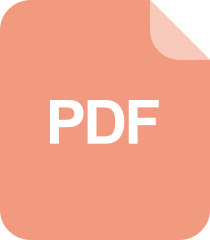
python爬取天气数据
首先,你需要安装一些库,比如requests、beautifulsoup4、pandas和matplotlib。你可以使用以下命令安装它们:
```
pip install requests beautifulsoup4 pandas matplotlib
```
然后,你需要从一个天气网站上爬取数据。这里我们以中国天气网为例,使用requests和BeautifulSoup来获取数据:
```python
import requests
from bs4 import BeautifulSoup
url = 'http://www.weather.com.cn/weather/101010100.shtml'
res = requests.get(url)
res.encoding = 'utf-8'
soup = BeautifulSoup(res.text, 'html.parser')
```
接下来,我们需要从页面中提取出我们需要的数据,比如天气、温度、风力等。你可以使用Chrome浏览器的“检查”功能来查看页面中这些数据的位置和标签,然后使用BeautifulSoup来提取数据。
```python
# 获取城市名
city = soup.select('.crumbs a')[-1].text.strip()
# 获取天气信息
weather = [i.text.strip() for i in soup.select('.t .wea')]
# 获取温度信息
temp = [i.text.strip() for i in soup.select('.t .tem')]
# 获取风力信息
wind = [i.text.strip() for i in soup.select('.t .win')]
# 获取日期信息
date = [i.text.strip() for i in soup.select('.t .day')]
```
接下来,我们将这些数据存储在一个pandas的DataFrame中,并进行可视化。这里我们使用matplotlib库来绘制图表。
```python
import pandas as pd
import matplotlib.pyplot as plt
# 存储数据
data = pd.DataFrame({
'日期': date,
'天气': weather,
'温度': temp,
'风力': wind
})
# 绘制温度折线图
plt.plot(data['日期'], data['温度'])
plt.title(f'{city}未来5天温度变化')
plt.xlabel('日期')
plt.ylabel('温度(℃)')
plt.show()
```
这样就可以得到一个简单的温度折线图了。你可以根据需要继续优化和完善代码。
阅读全文
相关推荐

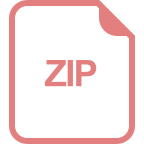
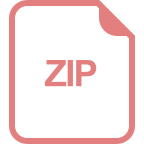
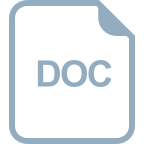
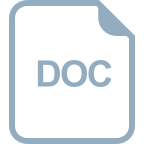




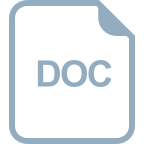
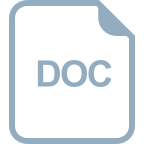



