计算曲线的一阶导数 python 示例代码
时间: 2023-07-25 19:13:06 浏览: 77
以下是一个用 Python 计算曲线一阶导数的示例代码:
```python
import sympy
# 定义变量和函数
x = sympy.Symbol('x')
f = x**2 + 2*x + 1
# 计算一阶导数
df = sympy.diff(f, x)
# 打印结果
print("f(x) =", f)
print("f'(x) =", df)
```
输出结果为:
```
f(x) = x**2 + 2*x + 1
f'(x) = 2*x + 2
```
其中,使用 `sympy` 库来定义变量和函数,并计算一阶导数。可以将 `f` 和 `df` 替换成需要计算的函数和导数。
相关问题
计算曲线的一阶导数 pandas
`pandas` 是一个用于数据处理和分析的 Python 库,它主要用于处理和分析数据集。它不适用于计算曲线的一阶导数。计算曲线的一阶导数需要使用数学库,如 `sympy` 或 `numpy`。前面我已经给出了 `sympy` 的示例代码,下面是一个使用 `numpy` 计算曲线一阶导数的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义函数
def f(x):
return x**2 + 2*x + 1
# 定义 x 的范围
x = np.linspace(-5, 5, 100)
# 计算一阶导数
df = np.gradient(f(x), x)
# 绘制曲线和导数
plt.plot(x, f(x), label="f(x)")
plt.plot(x, df, label="f'(x)")
plt.legend()
plt.show()
```
输出结果为一张包含曲线和导数的图表。其中,使用 `numpy` 库的 `linspace` 函数来定义 `x` 的范围,并使用 `numpy` 库的 `gradient` 函数来计算一阶导数。可以将 `f` 替换成需要计算的函数。
红外光谱预处理一阶导数python
在Python中进行红外光谱预处理的一种常见方法是使用一阶导数。一阶导数可以帮助提取光谱数据中的细微变化和特征。
以下是使用Python进行红外光谱一阶导数预处理的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 假设有一组原始的红外光谱数据
raw_data = np.array([0.1, 0.3, 0.2, 0.4, 0.6, 0.5, 0.3, 0.2, 0.1])
# 计算一阶导数
first_derivative = np.gradient(raw_data)
# 绘制原始数据和一阶导数
plt.subplot(2, 1, 1)
plt.plot(raw_data)
plt.title('Raw Data')
plt.subplot(2, 1, 2)
plt.plot(first_derivative)
plt.title('First Derivative')
plt.tight_layout()
plt.show()
```
在这个示例中,我们首先定义了一组原始的红外光谱数据`raw_data`。然后,使用`np.gradient`函数计算了这组数据的一阶导数,并将结果存储在`first_derivative`中。最后,我们使用Matplotlib库绘制了原始数据和一阶导数的图形。
你可以根据自己的实际需求修改这个示例代码,并应用到你的红外光谱预处理项目中。
相关推荐
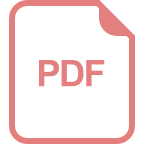












