CNN各个网络的pytorch代码
时间: 2024-09-21 15:10:15 浏览: 30
卷积神经网络(Convolutional Neural Network, CNN)在PyTorch中通常通过`torch.nn`模块来实现。下面是一个简单的例子,展示了如何创建一个基本的卷积层和全连接层组成的CNN模型:
```python
import torch
import torch.nn as nn
class SimpleCNN(nn.Module):
def __init__(self, input_channels, num_classes):
super(SimpleCNN, self).__init__()
# 卷积层
self.conv1 = nn.Conv2d(input_channels, 32, kernel_size=3, stride=1, padding=1)
self.relu1 = nn.ReLU() # 激活函数
self.pool1 = nn.MaxPool2d(kernel_size=2, stride=2) # 下采样
# 第二个卷积层
self.conv2 = nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1)
self.relu2 = nn.ReLU()
self.pool2 = nn.MaxPool2d(kernel_size=2, stride=2)
# 全连接层
self.fc1 = nn.Linear(64 * 8 * 8, 128) # 假设输入图片是8x8经过池化后的大小
self.relu_fc = nn.ReLU()
self.dropout = nn.Dropout(p=0.5) # 防止过拟合
self.fc2 = nn.Linear(128, num_classes)
def forward(self, x):
x = self.conv1(x)
x = self.relu1(x)
x = self.pool1(x)
x = self.conv2(x)
x = self.relu2(x)
x = self.pool2(x)
# 将张量展平并连接到全连接层
x = x.view(-1, 64 * 8 * 8)
x = self.fc1(x)
x = self.relu_fc(x)
x = self.dropout(x)
x = self.fc2(x)
return x
# 使用示例
model = SimpleCNN(3, 10) # 输入通道3(RGB),假设有10个类别
input_tensor = torch.randn(1, 3, 32, 32) # (batch_size, channels, height, width)
output = model(input_tensor)
```
这个例子中,我们创建了一个包含两个卷积层、两次池化操作以及两个全连接层的模型。每个部分都有相应的激活函数,如ReLU,以及在全连接层之前可能会有的Dropout层。
相关推荐
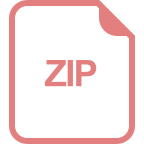
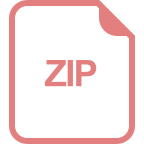
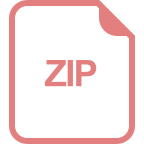
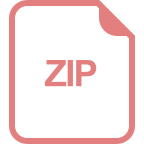
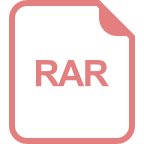
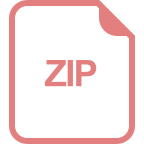
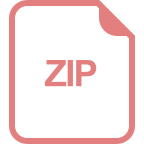
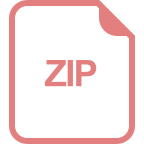
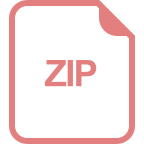
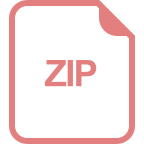
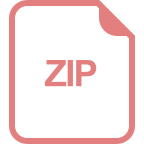
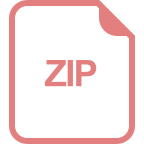
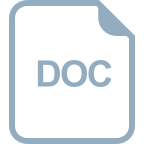
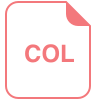
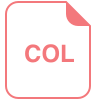
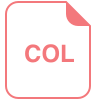
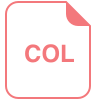
