c语言完全二叉树顺序存储代码
时间: 2024-11-11 08:14:26 浏览: 29
在C语言中,我们可以使用数组来表示完全二叉树,因为完全二叉树的特点是除了最后一层外,每一层都是满的,且最后一个节点都尽可能地靠左。以下是创建一个完全二叉树并按照顺序存储的简单代码示例:
```c
#include <stdio.h>
#define MAX_SIZE 50 // 定义最大节点数
typedef struct TreeNode {
int data; // 节点值
struct TreeNode* left;
struct TreeNode* right;
} Node;
// 创建一个空的二叉树节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->left = newNode->right = NULL;
return newNode;
}
// 插入节点到完全二叉树
void insertIntoCompleteTree(Node** root, int value, int size) {
if (*root == NULL || size == 0) {
*root = createNode(value);
return;
}
int index = size - 1;
Node* current = *root;
while (current != NULL && index >= 0) {
if (value <= current->data) {
if (index == 0) {
if (value < current->data) { // 如果新值小于当前值,插入左子树
current->left = createNode(value);
break;
} else {
current->left = NULL; // 否则将新值设为左孩子
break;
}
} else {
current = current->left;
index--;
}
} else {
if (index == 0) {
if (value > current->data) { // 如果新值大于当前值,插入右子树
current->right = createNode(value);
break;
} else {
current->right = NULL; // 否则将新值设为右孩子
break;
}
} else {
current = current->right;
index--;
}
}
}
}
// 打印完全二叉树
void printCompleteBST(Node* root) {
if (root == NULL) {
return;
}
printCompleteBST(root->left);
printf("%d ", root->data); // 先打印左子树,然后根节点,最后右子树
printCompleteBST(root->right);
}
int main() {
Node* root = NULL;
int nodes[] = {4, 2, 6, 1, 3, 5, 7};
int n = sizeof(nodes) / sizeof(nodes[0]);
for (int i = 0; i < n; i++) {
insertIntoCompleteTree(&root, nodes[i], n);
}
printf("Complete Binary Tree in order:\n");
printCompleteBST(root);
return 0;
}
```
这段代码首先定义了一个二叉树结构体`TreeNode`,接着实现了创建节点、插入节点和打印完整二叉树的函数。在`main`函数中,我们创建了一个完全二叉树,并按照顺序存储了给定的节点。
阅读全文
相关推荐
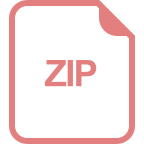
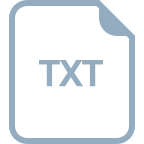
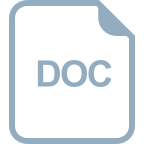
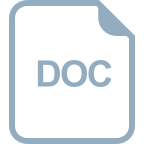
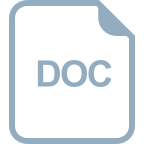








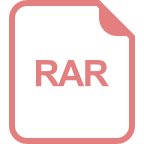
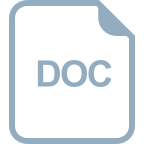
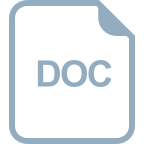
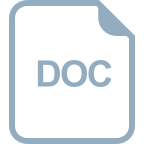

