c++顺时针输出二维数组
时间: 2023-12-17 21:24:50 浏览: 85
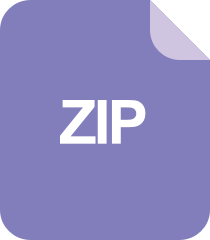
顺时针打印二维数组中的元素
要顺时针输出二维数组,可以按照以下步骤进行:
1. 初始化四个边界变量:top、bottom、left、right,分别表示当前输出的上边界、下边界、左边界和右边界。
2. 初始化一个变量dir,表示当前遍历的方向,初始值为0,表示向右遍历。
3. 使用一个循环,直到上边界大于下边界或左边界大于右边界为止:
- 如果dir为0,依次输出上边界的元素,然后将上边界下移一行,即top加1。
- 如果dir为1,依次输出右边界的元素,然后将右边界左移一列,即right减1。
- 如果dir为2,依次输出下边界的元素,然后将下边界上移一行,即bottom减1。
- 如果dir为3,依次输出左边界的元素,然后将左边界右移一列,即left加1。
- 每次输出完一个边界后,需要更新dir的值为(dir+1)%4,以改变遍历的方向。
4. 完成遍历后即可得到顺时针输出的结果。
以下是使用Python实现的代码示例:
```python
def clockwisePrint(matrix):
if not matrix:
return []
top, bottom = 0, len(matrix) - 1
left, right = 0, len(matrix[0]) - 1
dir = 0
res = []
while top <= bottom and left <= right:
if dir == 0:
for i in range(left, right + 1):
res.append(matrix[top][i])
top += 1
elif dir == 1:
for i in range(top, bottom + 1):
res.append(matrix[i][right])
right -= 1
elif dir == 2:
for i in range(right, left - 1, -1):
res.append(matrix[bottom][i])
bottom -= 1
else:
for i in range(bottom, top - 1, -1):
res.append(matrix[i][left])
left += 1
dir = (dir + 1) % 4
return res
```
阅读全文
相关推荐
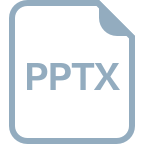
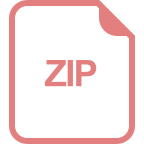
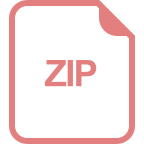
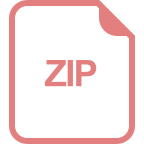
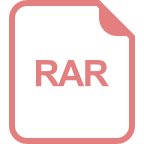
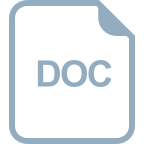
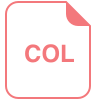









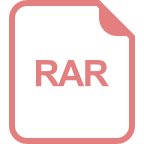