如何按照fragment的原理分成左右两个fragment,左边可以看成是一个广告fragment,把右边的RecyclerView封装到一个Fragment中,这样左右都是放在独立的Fragment中,求实验步骤
时间: 2024-10-22 21:09:37 浏览: 23
要在Android中按照Fragment的原理将屏幕分为左右两个部分,其中左侧显示广告,右侧是一个RecyclerView,你可以按以下步骤操作:
1. **创建基础布局**:
- 在`activity_main.xml`布局文件中,设置一个LinearLayout或者其他支持分隔布局的容器。例如:
```xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:id="@+id/left_ad_container"
android:layout_width="0dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintWidth_default="wrap"
android:layout_weight="1"
android:background="#F9F9F9" />
<FrameLayout
android:id="@+id/right_recycler_view_container"
android:layout_width="0dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintWidth_default="wrap"
android:layout_weight="3"
android:background="@color/your_recycler_view_color" />
</ConstraintLayout>
```
2. **创建广告Fragment**:
- 创建一个新的Fragment,比如`AdFragment.java`,并添加一个网络请求或加载静态数据的组件(如ImageView或WebView),展示广告内容。
3. **创建RecyclerView Fragment**:
- 创建另一个Fragment,比如`RecyclerViewFragment.java`,负责管理RecyclerView的数据源。可以使用Adapter和数据模型类,如`MyViewHolder`, `MyAdapter`, 和 `List<MyData>` 类型的数据列表。
4. **在Activity中管理和切换Fragment**:
- 在`MainActivity.java`或对应的BaseActivity中,通过FragmentManager管理这两个Fragment的切换。首先,将`AdFragment` 设置为初始可见的Fragment,然后在界面的某个按钮或监听事件触发时,替换当前显示的Fragment,例如:
```java
private void showLeftAd() {
getSupportFragmentManager()
.beginTransaction()
.replace(R.id.left_ad_container, new AdFragment())
.commit();
}
private void showRightRecyclerView() {
getSupportFragmentManager()
.beginTransaction()
.replace(R.id.right_recycler_view_container, new RecyclerViewFragment())
.commit();
}
```
这里假设`showLeftAd()` 显示广告Fragment,`showRightRecyclerView()` 显示RecyclerView Fragment。
5. **传递数据或通信**:
- 如果需要在两个Fragment之间传递数据,可以考虑使用接口、EventBus、LiveData等方案。如果广告Fragment影响到了RecyclerView Fragment的行为,可能需要使用观察者模式或者传入事件参数。
阅读全文
相关推荐
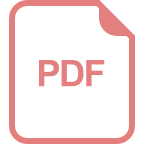
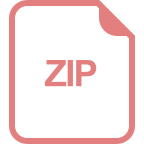
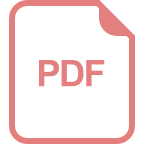
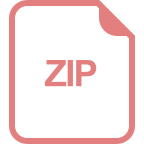
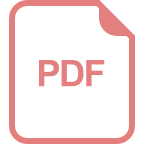
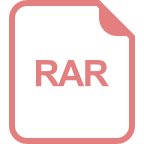
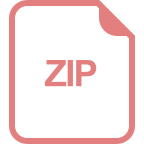
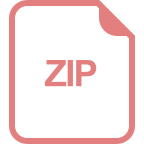
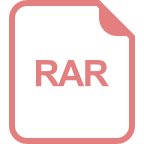
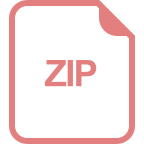
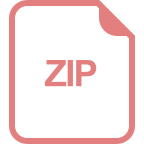
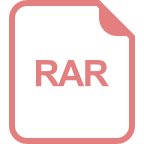
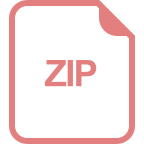
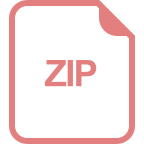
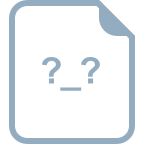
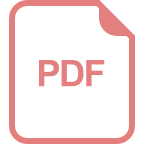
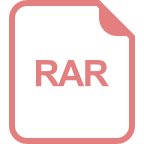
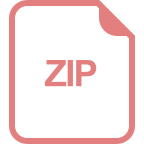