栅格 a星 matlab
时间: 2023-11-30 08:01:11 浏览: 98
栅格 A* 是一种用于路径规划的算法,在 MATLAB 中可以使用该算法来寻找起点到终点之间的最佳路径。该算法结合了Dijkstra算法和启发式搜索算法,通过在栅格地图上搜索,找到一条最优的路径。
在 MATLAB 中,可以通过创建栅格地图,并指定起点和终点的位置,然后使用 A* 算法来计算出最佳路径。算法会考虑到每个栅格的代价和启发式函数的估计值,从而找到一条最佳的路径。
使用 MATLAB 中的 A* 算法,可以通过指定一些参数来调整算法的行为,比如指定栅格地图的大小、代价函数、启发式函数等。通过调整这些参数,可以得到不同的路径规划结果,从而满足不同的应用需求。
除了路径规划之外,栅格 A* 算法还可以在 MATLAB 中应用于机器人导航、自动驾驶、游戏开发等领域。通过结合 MATLAB 提供的其他功能,比如图形显示和数据处理,可以将栅格 A* 算法应用到更广泛的应用中。
总之,栅格 A* 算法在 MATLAB 中具有广泛的应用前景,可以用于解决各种路径规划和导航问题,为各种应用提供优质的路径规划解决方案。
相关问题
a星算法栅格地图matlab
A星算法是一种在栅格地图中寻找最优路径的算法。在matlab中,可以利用矩阵表示栅格地图,每个格子代表一个节点,节点之间的连接代表路径的通行情况。利用A星算法,可以在这个栅格地图中找到起点到终点的最优路径。
首先,需要在matlab中定义栅格地图,并将起点和终点的坐标标注出来。然后,利用A星算法对栅格地图进行搜索,找到起点到终点的最优路径。在搜索过程中,A星算法会根据启发式函数来评估节点的优先级,以确定下一个需要扩展的节点。这个启发式函数可以是节点到终点的预估距离,也可以是节点到起点的真实距离。
在搜索过程中,A星算法会逐步扩展节点,并更新节点的优先级,直到找到终点或者无法再扩展节点为止。最终,A星算法会返回起点到终点的最优路径。
在matlab中,可以利用图形界面来显示栅格地图和最优路径,便于直观观察和验证算法的结果。通过这种方法,可以在matlab中实现A星算法对栅格地图的路径规划,应用于各种领域,如无人机航迹规划、机器人导航等。
a星算法matlab栅格地图
以下是一个简单的A*算法的MATLAB代码示例,用于在栅格地图上搜索最短路径:
```matlab
% 定义地图和起点终点坐标
map = [0 0 0 0 0 0 0 0;
0 1 1 0 0 0 0 0;
0 0 1 1 0 0 0 0;
0 0 0 1 1 0 0 0;
0 0 0 0 1 1 0 0;
0 0 0 0 0 1 0 0;
0 0 0 0 0 1 1 0;
0 0 0 0 0 0 0 0];
start_coords = [3, 1];
end_coords = [6, 8];
% A*算法
[route, numExpanded] = AStarGrid(map, start_coords, end_coords);
% 展示结果
imagesc(map);
hold on;
plot(start_coords(2), start_coords(1), 'gx');
plot(end_coords(2), end_coords(1), 'rx');
plot(route(:,2), route(:,1), 'b', 'LineWidth', 2);
```
其中,AStarGrid函数实现了A*算法:
```matlab
function [route,numExpanded] = AStarGrid (input_map, start_coords, end_coords)
% 八个邻居的相对坐标
delta = [[-1, 0]; % 上
[ 0,-1]; % 左
[ 1, 0]; % 下
[ 0, 1]; % 右
[-1,-1]; % 左上
[-1, 1]; % 右上
[ 1,-1]; % 左下
[ 1, 1]]; % 右下
% 获取地图的大小和起点终点坐标
[numrows, numcols] = size(input_map);
start_node = sub2ind([numrows, numcols], start_coords(1), start_coords(2));
end_node = sub2ind([numrows, numcols], end_coords(1), end_coords(2));
% 初始化启发函数值和代价函数值
h = heuristic(start_node, end_node, numcols);
g = Inf(numrows, numcols);
f = Inf(numrows, numcols);
% 初始化Open和Closed列表
closed_list = zeros(numrows, numcols);
open_list = [start_node; h(start_node)];
count = 0;
path_found = false;
% 开始搜索
while ~isempty(open_list)
% 获取Open列表中启发函数值最小的节点
[~, current] = min(open_list(2,:));
% 如果已经到达终点,则结束搜索
if current == end_node
path_found = true;
break;
end
% 将当前节点从Open列表中移除并加入Closed列表
open_list(:,current) = [];
closed_list(current) = 1;
count = count + 1;
% 获取当前节点的邻居节点
neighbors = bsxfun(@plus, delta, [floor((current-1)/numrows), mod(current-1,numrows)])';
neighbors = neighbors(:, all(neighbors >= 1 & bsxfun(@le, neighbors, [numcols, numrows])', 1));
for neighbor = neighbors
% 如果邻居节点已经在Closed列表中,则忽略
if closed_list(sub2ind([numrows, numcols], neighbor(1), neighbor(2))) == 1
continue;
end
% 如果邻居节点是障碍,则忽略
if input_map(neighbor(1), neighbor(2)) ~= 0
continue;
end
% 计算邻居节点的代价函数值
tentative_g = g(current) + norm(neighbor - [floor((current-1)/numrows), mod(current-1,numrows)]) + 1;
% 如果邻居节点不在Open列表中,则加入
neighbor_index = sub2ind([numrows, numcols], neighbor(1), neighbor(2));
if isempty(find(open_list(1,:) == neighbor_index, 1))
h(neighbor_index) = heuristic(neighbor_index, end_node, numcols);
open_list = [open_list, [neighbor_index; tentative_g+h(neighbor_index)]];
elseif tentative_g >= g(neighbor_index)
continue;
end
% 更新邻居节点的代价函数值和启发函数值
g(neighbor_index) = tentative_g;
f(neighbor_index) = tentative_g + h(neighbor_index);
end
end
% 如果找到了路径,则返回路径和扩展节点数
if path_found
route = reconstruct_path(closed_list, start_node, end_node, numrows);
numExpanded = count;
else
route = [];
numExpanded = 0;
end
end
% 计算启发函数值
function h = heuristic(current, goal, numcols)
[y, x] = ind2sub([numcols, numcols], current);
[gy, gx] = ind2sub([numcols, numcols], goal);
h = norm([x - gx, y - gy]);
end
% 重构路径
function path = reconstruct_path(closed_list, start_node, end_node, numrows)
path = [end_node];
while path(1) ~= start_node
[y, x] = ind2sub([numrows, numrows], path(1));
neighbors = [y-1, x; y, x-1; y+1, x; y, x+1; y-1, x-1; y-1, x+1; y+1, x-1; y+1, x+1];
neighbors = neighbors(all(neighbors >= 1 & bsxfun(@le, neighbors, [numrows, numrows]), 2),:);
[~, idx] = min(sum(bsxfun(@minus, neighbors, ind2sub([numrows, numrows], path(1))).^2, 2));
path = [sub2ind([numrows, numrows], neighbors(idx,1), neighbors(idx,2)); path];
end
end
```
该代码实现了一个简单的A*算法,以找到从起点到终点的最短路径。在执行代码之前,您需要将地图和起点/终点坐标传递给算法。其中,地图是一个二维数组,其中0表示可行区域,1表示障碍物。起点和终点坐标是一个长度为2的数组,表示它们在地图中的行列坐标。当算法完成后,它将返回找到的路径和扩展节点数。您可以通过绘制地图和路径来可视化结果。
阅读全文
相关推荐
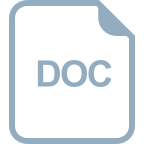
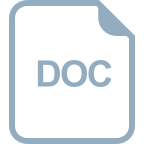
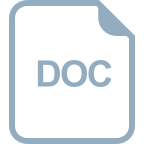
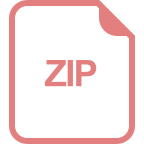
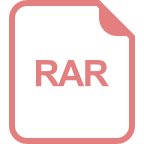
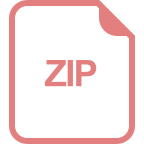
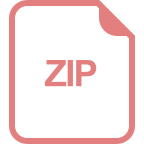
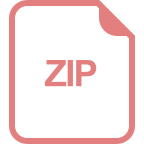
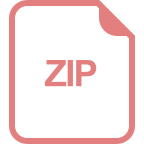
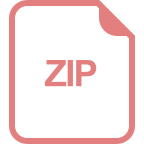
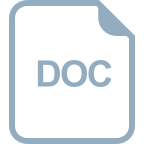
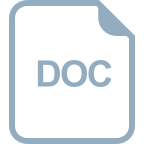
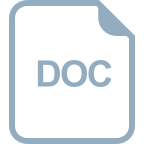
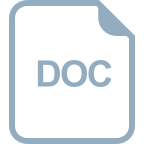