yuv数据编码H264并实时封装为ps流 c++ 程序
时间: 2024-09-28 21:14:13 浏览: 2
在C++中,要实现实时将YUV数据编码成H.264 (MPEG-4 Part 10) 视频流并封装为PS(PlayStation)流,你需要使用一些专门处理视频编码的库,比如FFmpeg或者OpenH264。以下是大致步骤:
1. **安装FFmpeg库**:FFmpeg是一个广泛使用的开源库,它包含了众多的音频和视频处理工具,包括H264编码。
```cpp
#include <ffmpeg.h>
```
2. **初始化FFmpeg上下文**:
```cpp
av_register_all();
avformat_network_init();
AVFormatContext* fmt_ctx = avformat_alloc_context();
```
3. **设置输出格式**:
```cpp
char filename[] = "output.mp4"; // 输出文件名
fmt_ctx->oformat = av_guess_format("mp4", filename, NULL);
if (!fmt_ctx->oformat)
av_log(NULL, AV_LOG_ERROR, "Could not guess output format.\n", 0);
```
4. **打开输出文件**:
```cpp
if (avio_open(&fmt_ctx->pb, filename, AVIO_FLAG_WRITE) < 0) {
av_log(NULL, AV_LOG_FATAL, "Failed to open file for writing\n", 0);
}
```
5. **添加流**:
```cpp
AVCodecContext* codec_ctx;
codec_ctx = avcodec_alloc_context3(NULL);
codec_ctx->codec_id = AV_CODEC_ID_H264;
// 配置H264编码器参数
AVStream* video_stream = avformat_new_stream(fmt_ctx, codec_ctx);
if (!video_stream) {
av_log(NULL, AV_LOG_FATAL, "Failed to add stream\n", 0);
}
video_stream->time_base = {1, fmt_ctx->r_frame_rate.num, fmt_ctx->r_frame_rate.den};
```
6. **编码和写入YUV数据**:
使用`avcodec_encode_video2()`函数进行编码,并写入到输出文件中。
7. **关闭和销毁资源**:
```cpp
av_write_trailer(fmt_ctx);
avcodec_free_context(&codec_ctx);
avformat_close_input(&fmt_ctx);
av_register_all(); // 可能需要再次注册以释放动态链接库
```
注意这只是一个基本框架,实际过程中还需要处理错误检查,可能需要调整编码参数以适应具体的YUV数据,以及根据需求选择合适的帧率、分辨率等配置。另外,实时流媒体可能涉及到多线程和解码缓冲等问题,你可能需要使用FFmpeg的RTSP或UDP推流功能,或者第三方库如Gstreamer来实现真正的实时传输。
相关推荐
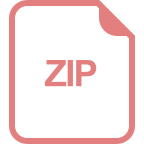
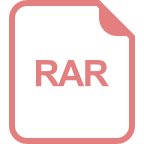
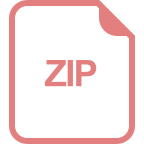














