按日期排序,不用函数来编写C语言代码
时间: 2024-12-08 09:19:52 浏览: 7
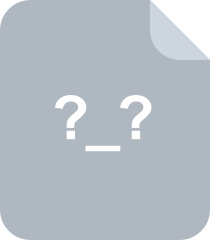
使用C语言编写的快速排序代码示例
在C语言中,如果你想要按照日期对一组数据进行排序,但是又不想直接使用标准库提供的排序函数如`qsort`或`sort`,你可以选择手动编写冒泡排序、选择排序、插入排序等简单的排序算法,这里以冒泡排序为例来演示如何按日期(假设我们有一个包含结构体`struct Date`的数组)来进行排序:
```c
#include <stdio.h>
#include <string.h>
// 假设Date结构如下,包含了年、月、日字段
typedef struct {
int year;
int month;
int day;
} Date;
// 比较两个日期大小的辅助函数
int compareDates(const void *a, const void *b) {
const Date *date1 = (const Date *)a;
const Date *date2 = (const Date *)b;
if (date1->year < date2->year)
return -1;
else if (date1->year > date2->year)
return 1;
else if (date1->month < date2->month)
return -1;
else if (date1->month > date2->month)
return 1;
else // 同年的同月份比较天数
return date1->day - date2->day;
}
void bubbleSortDates(Date dates[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - 1 - i; j++) {
if (compareDates(&dates[j], &dates[j + 1]) > 0) {
// 交换元素
Date temp = dates[j];
dates[j] = dates[j + 1];
dates[j + 1] = temp;
}
}
}
}
int main() {
Date dates[] = { {2023, 1, 1}, {2022, 12, 31}, {2023, 2, 5} };
int n = sizeof(dates) / sizeof(dates[0]);
bubbleSortDates(dates, n);
printf("Sorted dates:\n");
for (int i = 0; i < n; i++) {
printf("%d-%02d-%02d\n", dates[i].year, dates[i].month, dates[i].day);
}
return 0;
}
```
在这个例子中,我们首先定义了一个自定义的比较函数`compareDates`,然后在`bubbleSortDates`函数中使用这个函数进行比较并进行冒泡排序。
阅读全文
相关推荐
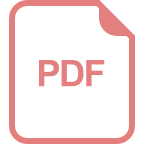
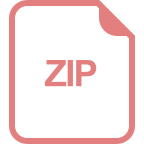
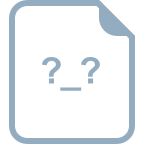
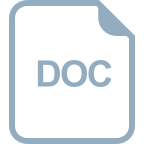
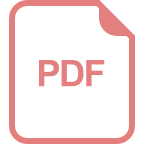
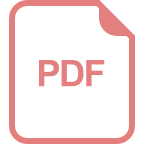
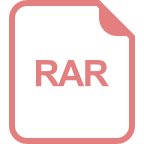
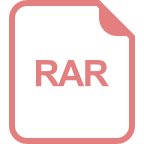
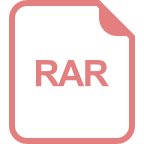
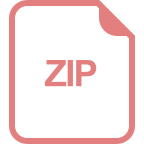
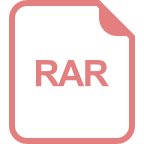
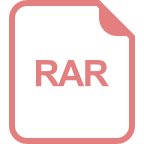
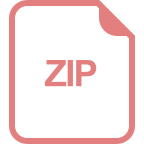
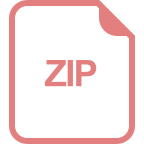
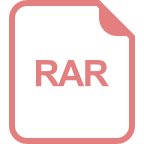
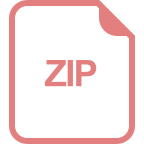