写出Java10个【非检查】异常的代码实例
时间: 2024-10-24 12:13:29 浏览: 10
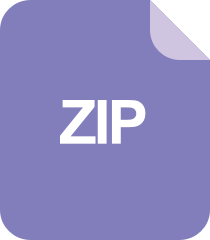
Java 实例 - 异常处理方法源代码-详细教程.zip
在Java中,非检查(Unchecked)异常也称为运行时异常,它们不是强制程序员处理的,因为它们通常表示程序逻辑错误而不是外部资源问题。以下是10个常见的非检查异常的例子,每个例子包含一个简短的代码片段:
1. `NullPointerException` (空指针异常)
```java
public class Main {
public static void main(String[] args) {
String str = null;
System.out.println(str.length());
}
}
```
2. `ArithmeticException` (算术异常)
```java
public class Main {
public static void main(String[] args) {
int a = 10;
int b = 0;
System.out.println(a / b);
}
}
```
3. `ArrayIndexOutOfBoundsException` (数组下标越界异常)
```java
public class Main {
public static void main(String[] args) {
int[] array = new int[5];
System.out.println(array[10]);
}
}
```
4. `IllegalArgumentException` (无效参数异常)
```java
public class Main {
public static void main(String[] args) {
String invalidName = "";
validateName(invalidName);
}
public static void validateName(String name) {
if (name.isEmpty()) throw new IllegalArgumentException("Name cannot be empty");
}
}
```
5. `IOException` (输入/输出异常)
```java
public class Main {
public static void main(String[] args) {
try {
File file = new File("non_existent_file.txt");
BufferedReader reader = new BufferedReader(new FileReader(file));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
```
6. `SQLException` (数据库连接异常)
```java
public class Main {
public static void main(String[] args) {
try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password")) {
// Some SQL operation here that throws an exception
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
7. `RuntimeException` (运行时异常的超类)
```java
public class Main {
public static void main(String[] args) {
throw new RuntimeException("Custom runtime exception");
}
}
```
8. `NumberFormatException` (数字格式转换异常)
```java
public class Main {
public static void main(String[] args) {
try {
Integer.parseInt("abc");
} catch (NumberFormatException e) {
e.printStackTrace();
}
}
}
```
9. `IllegalStateException` (非法状态异常)
```java
public class Main {
private boolean isClosed = false;
public void close() {
if (isClosed) throw new IllegalStateException("Already closed");
isClosed = true;
}
}
```
10. `UnsupportedOperationException` (不支持的操作异常)
```java
public class Main {
public void methodNotSupported() {
throw new UnsupportedOperationException("Method not supported");
}
}
```
阅读全文
相关推荐
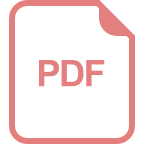
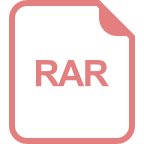


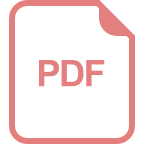
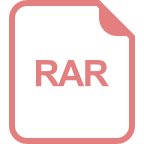
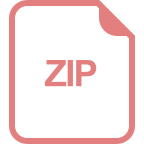
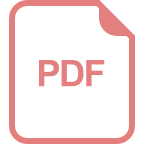
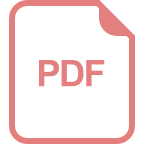
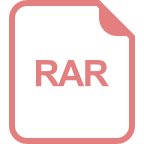
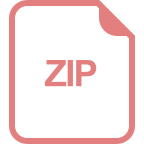
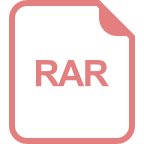
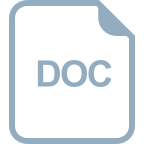



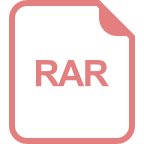
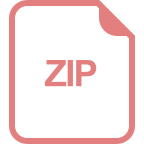