用fetch向php传参
时间: 2023-07-27 13:33:21 浏览: 59
在使用 Fetch API 向 PHP 传参时,需要使用 POST 请求,并将参数附加在请求的 body 中。以下是一个简单的示例:
```javascript
const data = {
name: 'John',
email: 'john@example.com'
};
fetch('example.php', {
method: 'POST',
body: JSON.stringify(data)
}).then(response => {
console.log(response);
});
```
上面代码中,我们将一个包含 name 和 email 参数的对象 data 通过 POST 请求发送到 example.php 文件中。在请求中,我们通过 JSON.stringify() 方法将 data 对象转换成 JSON 字符串,然后将其附加在请求的 body 中。在 PHP 中可以使用上面提到的方法来接收这个请求并获取参数。
需要注意的是,如果你没有在请求头中设置 Content-Type 为 application/json,PHP 默认会将请求体作为 application/x-www-form-urlencoded 类型处理。此时,你需要使用 $_POST 来获取参数,或者使用 parse_str() 方法将请求体解析成数组,例如:
```php
// 使用 $_POST 获取参数
$name = $_POST['name'];
$email = $_POST['email'];
// 或者使用 parse_str() 方法
parse_str(file_get_contents("php://input"), $data);
$name = $data['name'];
$email = $data['email'];
```
如果你希望将请求体以 JSON 格式传递到 PHP 中,需要在请求头中显式地设置 Content-Type 为 application/json,例如:
```javascript
const data = {
name: 'John',
email: 'john@example.com'
};
fetch('example.php', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
}).then(response => {
console.log(response);
});
```
这样,在 PHP 中就可以使用 file_get_contents('php://input') 方法获取原始请求体,并使用 json_decode() 方法解析 JSON,例如:
```php
$json = file_get_contents('php://input');
$data = json_decode($json);
$name = $data->name;
$email = $data->email;
```
相关推荐
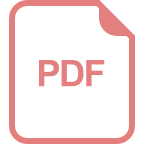
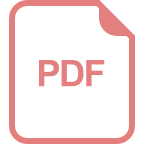
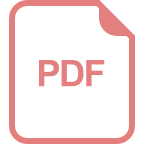














