微信小程序获取openid使用springsecurity和jwt实现权限验证过程和代码实现
时间: 2023-03-05 15:55:30 浏览: 92
使用 Spring Security 和 JWT 来实现微信小程序获取 OpenID 的权限验证过程和代码实现,首先要定义一个用于获取 OpenID 的过滤器,然后实现该过滤器,在过滤器中调用微信小程序的接口获取用户的 OpenID,然后将 OpenID 存储到 JWT 中,最后将 JWT token 返回给前端。
相关问题
微信小程序获取 openid
要获取微信小程序的openid,需要进行如下步骤:
1. 在微信公众平台中创建小程序,并获取小程序的AppID和AppSecret。
2. 在小程序中使用wx.login()函数获取用户的code,然后将code发送给后端服务器。
3. 后端服务器使用code和小程序的AppID和AppSecret向微信服务器发送请求,获取用户的openid。
4. 将获取到的openid返回给小程序,以便进行后续的业务逻辑处理。
需要注意的是,获取openid的过程需要涉及到小程序、后端服务器以及微信服务器之间的交互,因此需要在小程序和后端服务器中都进行相应的开发和配置。
微信小程序获取openId
要在微信小程序中获取用户的 OpenID,你可以通过调用微信登录接口来实现。以下是一个简单的示例代码:
1. 在小程序的相关页面或组件中引入微信登录 API:
```javascript
// 在页面或组件的js文件中引入微信登录 API
const app = getApp(); // 获取小程序实例
// 调用微信登录接口获取用户的 OpenID
wx.login({
success: res => {
if (res.code) {
// 发送 res.code 到后台换取 openId, sessionKey, unionId
wx.request({
url: 'https://api.weixin.qq.com/sns/jscode2session',
data: {
appid: app.globalData.appId, // 小程序的 AppID
secret: app.globalData.appSecret, // 小程序的 AppSecret
js_code: res.code,
grant_type: 'authorization_code'
},
success: res => {
console.log(res.data);
// 在这里可以获取到用户的 openId
const openId = res.data.openid;
// 将 openId 存储在本地或发送到服务器进行处理
},
fail: err => {
console.error(err);
}
});
} else {
console.error('微信登录失败:' + res.errMsg);
}
},
fail: err => {
console.error(err);
}
});
```
2. 在小程序的 app.js 文件中,将 AppID 和 AppSecret 存储在全局变量中:
```javascript
App({
onLaunch: function () {
// 将小程序的 AppID 和 AppSecret 存储在全局变量中
this.globalData = {
appId: 'YourAppID',
appSecret: 'YourAppSecret'
};
}
});
```
请注意,获取 OpenID 需要在小程序的后台服务器进行处理,你需要在服务器端获取到微信返回的 sessionKey 和 openId,并进行相应的处理。上述代码仅仅是在小程序中获取到了 OpenID,你还需要将 OpenID 发送到服务器端进行进一步的处理。
相关推荐
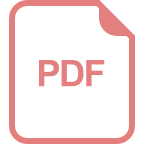
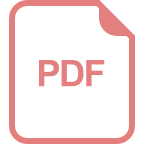
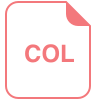
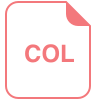
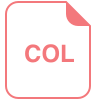
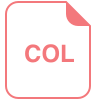
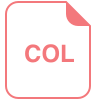







