编写一个c语言程序,以单链表的首结点值x为基准将该单链表分割为两个部分,使所有小于x的结点排在大于或等于x的结点之前
时间: 2024-12-14 17:19:04 浏览: 11
编写C语言程序来分割单链表可以根据给定的节点值`x`将链表分为两部分。这里是一个简单的示例,我们将创建一个函数`splitList`,它接受链表的头节点`head`以及基准值`x`作为输入:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
// 插入新节点到链表
ListNode* insertNode(ListNode *head, int val) {
ListNode *new_node = (ListNode*)malloc(sizeof(ListNode));
new_node->val = val;
new_node->next = head;
return new_node;
}
// 比较并分割链表
void splitList(ListNode **head, int x) {
ListNode *smaller_head = NULL, *smaller_tail = NULL;
ListNode *greater_head = head, *current = *head;
while (current != NULL) {
if (current->val < x) {
if (!smaller_head) {
smaller_head = current;
smaller_tail = current;
} else {
smaller_tail->next = current;
smaller_tail = current;
}
} else {
if (!greater_head) {
greater_head = current;
} else {
(*greater_head)->next = current;
}
}
current = current->next;
}
// 将小链表连接到大链表前
if (smaller_head) {
smaller_tail->next = greater_head;
*head = smaller_head;
} else {
*head = greater_head;
}
}
// 打印链表
void printList(ListNode *head) {
ListNode *temp = head;
while (temp != NULL) {
printf("%d ", temp->val);
temp = temp->next;
}
printf("\n");
}
int main() {
// 创建一个测试链表
ListNode *head = insertNode(NULL, 5);
insertNode(head, 2);
insertNode(head, 8);
insertNode(head, 1);
insertNode(head, 9);
int x = 5;
printf("Original List: ");
printList(head);
splitList(&head, x);
printf("Split List with %d as pivot: \n", x);
printList(head);
return 0;
}
```
在这个例子中,我们首先创建了一个单链表,然后调用`splitList`函数根据`x=5`分割链表。之后打印原始链表和分割后的链表。
阅读全文
相关推荐
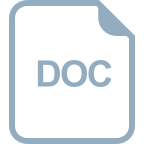
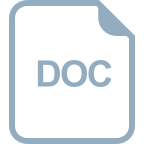
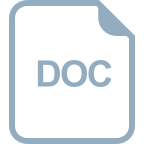
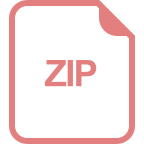
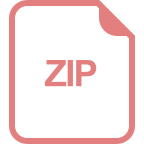
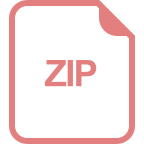
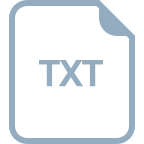
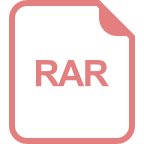
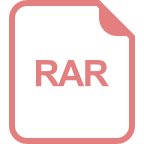
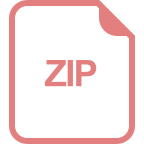
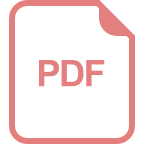
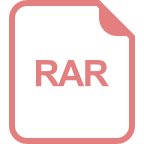
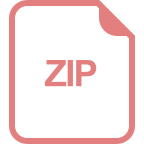
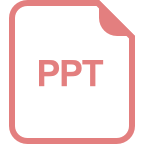