python 接口自动化测试脚本demo
时间: 2023-05-04 18:04:18 浏览: 169
Python 接口自动化测试脚本demo 是一个用 Python 编写的示例程序,用于演示如何使用 Python 来进行接口自动化测试。
该示例程序包括以下功能:
1. 读取 Excel 文件中的测试用例数据,并将其转换为 Python 对象。
2. 使用 requests 库发送 HTTP 请求,并获取响应。
3. 解析响应,提取关键信息并进行断言。
4. 将测试结果写入 Excel 文件中。
使用该示例程序进行接口自动化测试时,需要下载并安装 Python 3.x、requests 库和 openpyxl 库。并需修改程序中的 Excel 文件路径和请求地址等参数,以适应实际测试环境。
使用 Python 进行接口自动化测试,具有简单易学、灵活性高、可扩展性强等优点。相比其他测试工具,Python 编写的接口自动化测试脚本可以自定义实现更加复杂的测试场景,更具协同性和可维护性。因此,Python 接口自动化测试脚本是目前越来越多企业选择的测试方式。
相关问题
python自动化测试demo
好的,以下是一个简单的Python自动化测试demo,使用了unittest测试框架和Selenium WebDriver。
假设我们要测试一个简单的登录页面,包括用户名、密码和登录按钮。我们需要编写一个测试脚本,使用Selenium WebDriver进行自动化测试,并使用unittest框架进行测试管理和报告生成。
首先,我们需要安装Selenium WebDriver和unittest框架,以及引入它们的库:
```python
import unittest
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
```
然后,我们可以编写一个测试类,继承unittest.TestCase类,并在其中编写测试方法。例如,我们可以编写一个测试登录的方法:
```python
class TestLogin(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
self.driver.get("http://example.com/login")
def test_login(self):
user = self.driver.find_element_by_name("username")
user.send_keys("myusername")
password = self.driver.find_element_by_name("password")
password.send_keys("mypassword")
login_button = self.driver.find_element_by_xpath("//button[@type='submit']")
login_button.click()
welcome_message = self.driver.find_element_by_xpath("//div[@class='welcome-message']")
self.assertEqual(welcome_message.text, "Welcome, myusername!")
def tearDown(self):
self.driver.quit()
```
在测试方法中,我们首先打开浏览器并访问登录页面,然后输入用户名和密码并单击登录按钮。最后,我们检查是否出现了欢迎消息,并使用self.assertEqual方法进行断言。
最后,我们可以编写一个测试运行器,使用unittest.TestLoader和unittest.TextTestRunner运行测试并生成测试报告:
```python
if __name__ == '__main__':
loader = unittest.TestLoader()
suite = loader.loadTestsFromTestCase(TestLogin)
runner = unittest.TextTestRunner(verbosity=2)
runner.run(suite)
```
完整的测试脚本如下所示:
```python
import unittest
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
class TestLogin(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
self.driver.get("http://example.com/login")
def test_login(self):
user = self.driver.find_element_by_name("username")
user.send_keys("myusername")
password = self.driver.find_element_by_name("password")
password.send_keys("mypassword")
login_button = self.driver.find_element_by_xpath("//button[@type='submit']")
login_button.click()
welcome_message = self.driver.find_element_by_xpath("//div[@class='welcome-message']")
self.assertEqual(welcome_message.text, "Welcome, myusername!")
def tearDown(self):
self.driver.quit()
if __name__ == '__main__':
loader = unittest.TestLoader()
suite = loader.loadTestsFromTestCase(TestLogin)
runner = unittest.TextTestRunner(verbosity=2)
runner.run(suite)
```
这是一个简单的Python自动化测试demo,可以帮助您入门。当然,在实际项目中,您需要编写更复杂的测试脚本,并使用更多的Selenium和unittest功能来测试不同的场景和功能。
用python写一个接口自动化的demo脚本
以下是一个简单的接口自动化示例脚本,使用Python编写:
```python
import requests
# 接口地址
url = "https://jsonplaceholder.typicode.com/posts"
# 请求头信息
headers = {
'Content-Type': 'application/json'
}
# 请求体信息
data = {
"title": "foo",
"body": "bar",
"userId": 1
}
# 发送POST请求
response = requests.post(url, headers=headers, json=data)
# 打印响应信息
print(response.status_code)
print(response.json())
```
这个脚本使用了requests库来发送POST请求,向一个示例接口地址发送了请求体数据,并打印出了响应状态码和响应信息。可以根据实际需求修改接口地址、请求头和请求体信息。
阅读全文
相关推荐
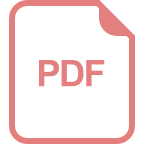
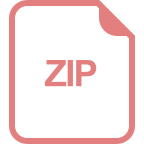
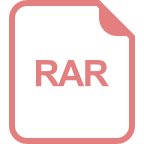
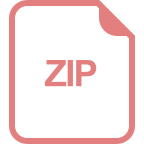
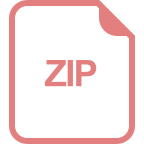
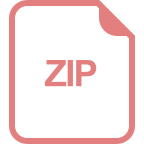
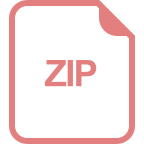
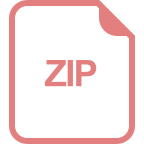
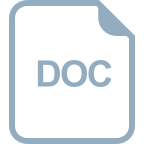
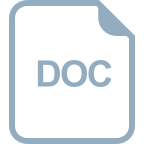
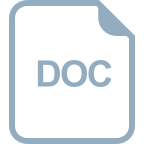
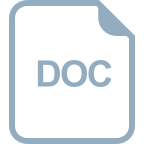
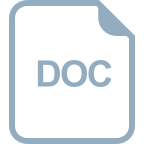
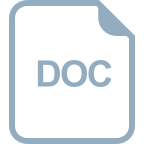