Linux进程调度有关函数的源代码
时间: 2023-11-18 08:03:02 浏览: 167
Linux进程调度有关的函数主要包括以下几个:
1. schedule():这个函数是Linux内核中最重要的进程调度函数之一,它会根据进程的优先级和调度策略来选择下一个要执行的进程,并将当前进程切换出去。
```
void __sched schedule(void)
{
struct task_struct *prev, *next;
struct rq *rq;
int cpu;
cpu = smp_processor_id();
rq = cpu_rq(cpu);
prev = rq->curr;
schedule_debug(prev);
if (unlikely(prev->state == TASK_RUNNING)) {
/*
* The previous task running on this CPU was not
* properly scheduled-away. Set the timestamp for
* its delay accounting here so that it is charged
* for its full timeslice.
*/
rq->clock_task = prev;
update_rq_clock(rq);
}
next = pick_next_task(rq);
clear_tsk_need_resched(prev);
rq->skip_clock_update = 0;
if (likely(prev != next)) {
rq->nr_switches++;
rq->curr = next;
++*switch_count;
/*
* If switching to a new process, reset the
* time slice counter
*/
if (unlikely(prev->policy != SCHED_BATCH)) {
if (unlikely(!prev->array)) {
prev->array = rq->active;
prev->array->nr_active++;
}
if (prev->se.exec_start == 0)
prev->se.exec_start = rq_clock_task(rq);
else if (task_cpu(prev) != cpu)
prev->se.exec_start = rq_clock_task(rq);
if (unlikely(++prev->se.nr_cpus_pin > 1))
prev->se.nr_cpus_pin = 1;
/*
* The running process is the last
* to have run on this cpu
*/
prev->cpu_timers.cpu = cpu;
prev->cpu_timers.prev_count = 0;
prev->cpu_timers.cur_clock = 0;
prev->cpu_timers.start_time = rq_clock_task(rq);
prev->state = TASK_RUNNING;
/*
* Use a barrier to ensure that the above
* stores complete before the stores to
* prev->on_cpu and rq->curr.
*/
smp_mb();
prev->on_cpu = cpu;
context_switch(rq, prev, next);
/*
* If the task got requeued meanwhile (e.g. signals),
* we don't want to lose track of it.
*/
if (prev->state == TASK_RUNNING)
resched_curr(rq);
}
}
task_rq_unlock(rq, &flags);
}
```
2. pick_next_task():这个函数会在可运行队列中选择下一个要执行的进程,并返回其指针。
```
static inline struct task_struct *
pick_next_task(struct rq *rq)
{
const struct sched_class *class;
struct task_struct *p;
class = rq->curr->sched_class;
p = class->pick_next_task(rq, rq->curr, rq->nr_running);
if (!p)
p = idle_task(rq);
return p;
}
```
3. enqueue_task():这个函数会将一个进程添加到可运行队列中。
```
static inline void
enqueue_task(struct rq *rq, struct task_struct *p, int flags)
{
const struct sched_class *class;
class = p->sched_class;
class->enqueue_task(rq, p, flags);
}
```
4. dequeue_task():这个函数会将一个进程从可运行队列中移除。
```
static inline void
dequeue_task(struct rq *rq, struct task_struct *p, int flags)
{
const struct sched_class *class;
class = p->sched_class;
class->dequeue_task(rq, p, flags);
}
```
5. yield():这个函数会让当前进程主动放弃CPU,让其他进程有机会执行。
```
void __sched yield(void)
{
struct task_struct *p = current;
struct rq *rq = task_rq_lock(p, NULL);
/*
* If we are the only task in the runqueue, we do not want
* to yield the CPU, but instead want to continue executing.
*/
if (unlikely(rq->nr_running == 1))
goto out_unlock;
p->state = TASK_RUNNING;
resched_curr(rq);
out_unlock:
task_rq_unlock(rq, NULL);
}
```
以上是Linux进程调度相关函数的部分源代码,它们的具体实现可能因不同的版本和架构而有所差异。
阅读全文
相关推荐

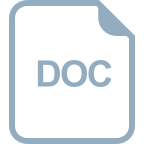
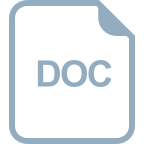
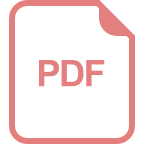
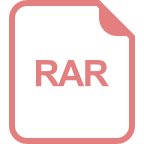
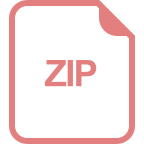
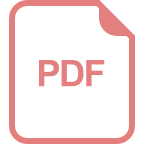
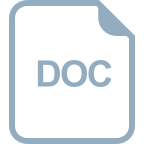
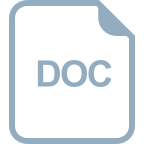

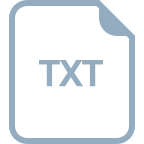
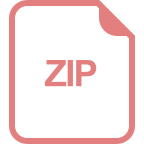
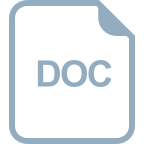
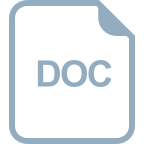
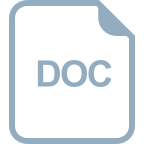